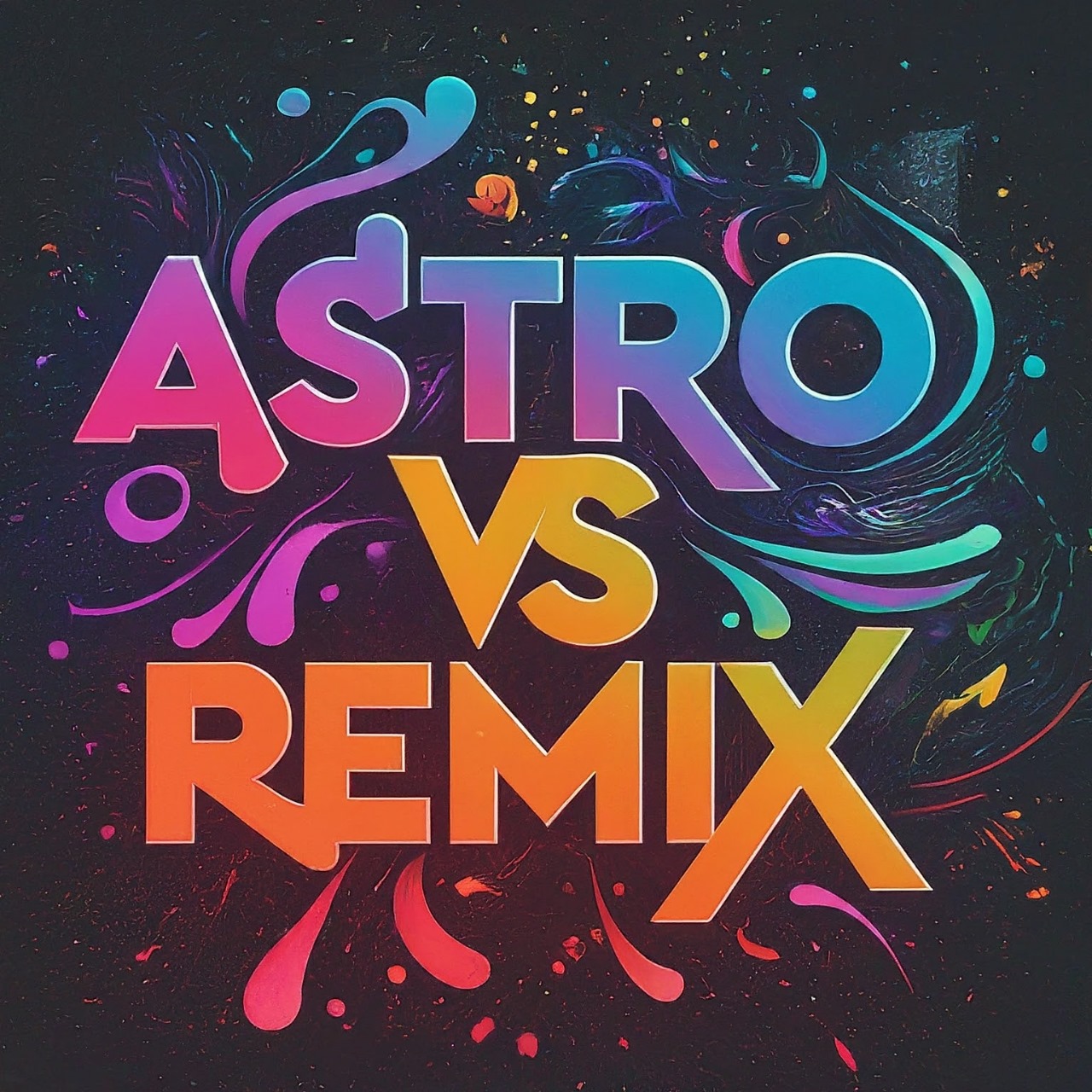
In the ever-evolving landscape of web development, React has established itself as a dominant force. Its component-based architecture, declarative nature, and vast ecosystem of tools have made it a top choice for building modern web applications. However, as projects grow in complexity, developers often seek out frameworks that provide additional structure, performance optimizations, and developer experience improvements. In this article, we’ll delve into two such frameworks: Remix and Astro.
Remix: Reinventing React Routing and Data Management
Remix is a full-stack React framework that reimagines how developers build web applications. It introduces a novel approach to routing and data management, aiming to simplify the development process while enhancing performance and maintainability.
Routing in Remix revolves around routes-as-components paradigm,where each route is defined as a React component.This approach offers several benefits:
- Declarative Routing: Routes are defined directly within the application’s component tree, making them easy to understand and maintain.
- Server-Rendered Routes: Remix seamlessly integrates server-side rendering (SSR) into the routing process, enabling faster page loads and improved SEO.
- Data Loading: Remix provides a robust data loading mechanism that allows developers to fetch data on the server or client before rendering a route, ensuring optimal performance and responsiveness.
Let’s take a look at a simple example of routing in Remix:
// app/routes/index.js
import { json } from 'remix';
export default function Index() {
return json({ message: 'Hello, Remix!' });
}
In this example, we define a basic route using a React component. The ‘json’ function is used to return JSON data, which can be rendered on the client or server depending on the context.
Data management in Remix is equally powerful, thanks to its built-in loader functions. These functions allow developers to fetch data asynchronously before rendering a route, ensuring that the page content is always up-to-date and consistent.
// app/routes/index.js
export let loader = async () => {
const data = await fetch('https://api.example.com/data');
return json(await data.json());
};
export default function Index({ data }) {
return <div>{data.message}</div>;
}
In this example, we use a loader function to fetch data from an API endpoint before rendering the route. The fetched data is then passed to the component as props, allowing for seamless integration with the rest of the application.
Overall, Remix offers a modern approach to building React applications, with a focus on simplicity, performance, and developer experience.
Astro: Blazing Fast Static Sites with React and Beyond
Astro takes a different approach to web development, aiming to combine the best of static site generation (SSG) and server-side rendering (SSR) into a single framework. It empowers developers to build fast, modern websites using familiar tools and technologies like React, Vue.js, and Svelte.
At the heart of Astro is its Astro Component System, which allows developers to build UI components using their framework of choice. These components can then be seamlessly integrated into Astro projects, enabling rapid development and easy collaboration among team members.
Let’s explore a simple example of building a React component in Astro:
// components/HelloWorld.astro
---
import React from 'react';
---
const HelloWorld = () => {
return <h1>Hello, Astro!</h1>;
};
export default HelloWorld;
In this example, we define a basic React component using JSX syntax. This component can then be used anywhere within an Astro project, providing a reusable building block for creating dynamic and interactive user interfaces.
Astro also introduces the concept of “partial hydration,” which allows developers to selectively hydrate components on the client-side based on user interactions or device capabilities. This can lead to significant performance improvements, especially on mobile devices with limited resources.
// components/Counter.astro
---
import { useState } from 'react';
---
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
export default Counter;
In this example, we define a simple counter component that uses React’s ‘useState’ hook to manage state locally. By selectively hydrating this component on the client-side, Astro ensures a smooth user experience while minimizing unnecessary JavaScript execution.
Conclusion:
In this article, we’ve explored two innovative frameworks for building React applications: Remix and Astro. While Remix focuses on reimagining routing and data management, Astro aims to combine the best of static site generation and server-side rendering. Both frameworks offer unique features and benefits, empowering developers to build fast, modern web applications with ease. Whether you’re looking for performance optimizations, developer experience improvements, or a combination of both, Remix and Astro have you covered. So why not give them a try and see how they can elevate your next React project.