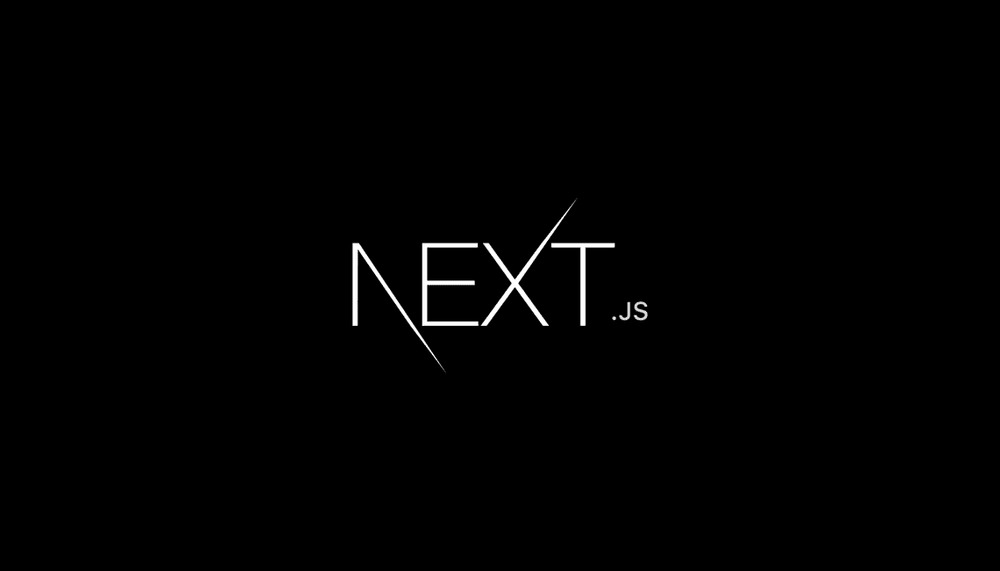
In the world of web development, frameworks play a pivotal role in simplifying the process of building robust, scalable, and efficient web applications. Among the myriad of options available, Next.js has emerged as a prominent player, offering developers a powerful toolkit for crafting modern web experiences. What sets Next.js apart is its unique meta framework approach, which combines the best features of server-side rendering (SSR), static site generation (SSG), and client-side rendering (CSR) into a single cohesive platform. In this comprehensive guide, we will delve deep into the meta framework of Next.js, exploring its core concepts, features, and practical applications.
Understanding the Meta Framework
At its core, Next.js is a React framework that allows developers to build dynamic web applications with ease. What makes Next.js truly innovative is its meta framework architecture, which seamlessly integrates server-side rendering, static site generation, and client-side rendering, providing developers with unparalleled flexibility and performance optimization.
Server-side Rendering (SSR)
Server-side rendering is a technique used to generate HTML pages on the server and send them to the client’s browser. This approach offers several advantages, including improved SEO, faster initial page loads, and better performance on low-powered devices. Next.js excels in SSR, thanks to its built-in support for server-side rendering out of the box. By simply creating a ‘getServerSideProps’ function in a page component, developers can fetch data from external APIs or databases and pass it directly to the component for rendering on the server.
// pages/blog/[slug].js
export async function getServerSideProps(context) {
const { params } = context;
const { slug } = params;
// Fetch data from external API
const postData = await fetch(`https://api.example.com/posts/${slug}`);
const postDataJson = await postData.json();
return {
props: {
postData: postDataJson,
},
};
}
function BlogPost({ postData }) {
return (
<div>
<h1>{postData.title}</h1>
<p>{postData.content}</p>
</div>
);
}
export default BlogPost;
Static Site Generation (SSG)
Static site generation involves pre-building HTML pages at build time, which can then be served to clients without the need for server-side processing. This approach offers significant performance benefits, as static assets can be cached and delivered to users with minimal latency. Next.js supports SSG through its ‘getStaticProps’ and ‘getStaticPaths’ functions, allowing developers to generate static pages dynamically based on data fetched from various sources.
// pages/blog/[slug].js
export async function getStaticPaths() {
// Fetch list of blog post slugs from CMS
const res = await fetch('https://api.example.com/posts');
const posts = await res.json();
// Generate paths for all posts
const paths = posts.map((post) => ({
params: { slug: post.slug },
}));
return { paths, fallback: false };
}
export async function getStaticProps({ params }) {
const { slug } = params;
// Fetch data for the specific post
const res = await fetch(`https://api.example.com/posts/${slug}`);
const postData = await res.json();
return {
props: {
postData,
},
};
}
function BlogPost({ postData }) {
return (
<div>
<h1>{postData.title}</h1>
<p>{postData.content}</p>
</div>
);
}
export default BlogPost;
Client-side Rendering (CSR)
Client-side rendering involves rendering web pages directly in the browser using JavaScript. While this approach offers interactivity and real-time updates, it can lead to slower initial page loads and reduced SEO performance. Next.js supports CSR through its client-side navigation capabilities, allowing developers to fetch data and render components dynamically on the client side without full-page reloads.
// components/Comments.js
import { useState, useEffect } from 'react';
function Comments({ postId }) {
const [comments, setComments] = useState([]);
useEffect(() => {
// Fetch comments for the specified post
fetch(`https://api.example.com/posts/${postId}/comments`)
.then((response) => response.json())
.then((data) => setComments(data));
}, [postId]);
return (
<div>
<h2>Comments</h2>
<ul>
{comments.map((comment) => (
<li key={comment.id}>{comment.text}</li>
))}
</ul>
</div>
);
}
export default Comments;
// pages/blog/[slug].js
import Comments from '../../components/Comments';
function BlogPost({ postData }) {
return (
<div>
<h1>{postData.title}</h1>
<p>{postData.content}</p>
<Comments postId={postData.id} />
</div>
);
}
export default BlogPost;
Practical Applications
The meta framework of Next.js opens up a world of possibilities for developers, enabling them to create highly optimized web applications that leverage the best of SSR, SSG, and CSR. Here are some practical applications of Next.js meta framework:
- E-commerce Platforms: Next.js can be used to build e-commerce platforms that require fast page loads, SEO optimization, and real-time updates. By combining SSR for product pages, SSG for static content, and CSR for dynamic components like shopping carts, developers can create seamless shopping experiences for users.
- Content Management Systems (CMS): Next.js is well-suited for building CMS platforms that need to handle a large volume of dynamic content. By utilizing SSR and SSG to generate static pages for blog posts, articles, and other content, developers can ensure fast and reliable performance while maintaining flexibility for dynamic content updates.
- Dashboards and Analytics Tools: Next.js can be used to build data visualization dashboards and analytics tools that require real-time data updates and interactive user interfaces. By leveraging CSR for dynamic chart rendering and server-side caching for data fetching, developers can create powerful analytics platforms that deliver insights in real time.
- Progressive Web Applications (PWAs): Next.js is an excellent choice for building progressive web applications that offer a native app-like experience in the browser. By combining SSR for initial page loads, service workers for offline support, and CSR for dynamic content updates, developers can create PWAs that are fast, reliable, and engaging.
Next.js is a robust meta framework that empowers developers to craft cutting-edge web applications with enhanced performance, scalability, and user engagement. Its unique amalgamation of server-side rendering (SSR), static site generation (SSG), and client-side rendering (CSR) capabilities revolutionizes the web development landscape, offering a comprehensive toolkit for tackling diverse project requirements.
With Next.js, developers can seamlessly integrate SSR to render pages on the server, improving SEO and initial load times. This feature proves invaluable, particularly for content-heavy websites or applications where search engine visibility and speed are paramount. Moreover, Next.js simplifies the process of implementing SSR through intuitive APIs like getServerSideProps, streamlining data fetching and rendering on the server.
Additionally, Next.js excels in SSG, enabling developers to pre-build pages at build time for lightning-fast performance and efficient resource utilization. By leveraging functions like getStaticProps and getStaticPaths, developers can dynamically generate static pages based on data from various sources, ensuring optimal scalability and SEO optimization. This capability is particularly beneficial for websites with frequently changing content or large datasets, as it minimizes server load and accelerates content delivery.
Furthermore, Next.js facilitates CSR for interactive, dynamic user experiences, seamlessly transitioning between client-side rendering and server-side rendering as needed. This flexibility allows developers to implement complex client-side logic while ensuring fast page transitions and real-time updates. By leveraging client-side navigation and data fetching techniques, developers can create immersive web applications with seamless transitions and responsive interfaces.
Whether you’re building an e-commerce platform, a content management system, or a data visualization dashboard, Next.js provides the versatility and performance optimization required to bring your vision to life. Its intuitive APIs, comprehensive documentation, and vibrant community support make it an ideal choice for developers of all skill levels. So, why delay? Embrace the power of Next.js today and unlock the full potential of web development.