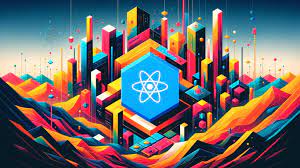
React, with its declarative and component-based approach, has revolutionized the way developers build web applications. One of the fundamental concepts in React is props.
props allow components to communicate with each other by passing data from parent to child. In this guide, we’ll delve deep into the world of React props, exploring its syntax, best practices, and real-world examples.
Understanding React Props
At its core, React props are a mechanism for passing data from one component to another. Props are immutable, meaning they cannot be modified within the component receiving them. This immutability ensures predictable behavior and makes debugging easier.
Syntax of Props
Let’s start with the syntax of props. Props are passed to a component as attributes in JSX. Here’s a simple example:
// ParentComponent.js
import React from 'react';
import ChildComponent from './ChildComponent';
function ParentComponent() {
return (
<div>
<ChildComponent name="John" age={30} />
</div>
);
}
export default ParentComponent;
In this example, we’re passing two props to the ‘ChildComponent’: ‘name’ and ‘age’. Now, let’s see how the ‘ChildComponent’ receives and uses these props:
// ChildComponent.js
import React from 'react';
function ChildComponent(props) {
return (
<div>
<p>Name: {props.name}</p>
<p>Age: {props.age}</p>
</div>
);
}
export default ChildComponent;
Here,’ props’ is an object containing all the properties passed to the component. We access individual props using dot notation, e.g., ‘props.name’ and ‘props.age’.
Default Props
React allows you to define default values for props using the defaultProps property. This is useful when certain props are not provided by the parent component. Let’s extend our previous example to include default props:
// ChildComponent.js
import React from 'react';
function ChildComponent(props) {
return (
<div>
<p>Name: {props.name}</p>
<p>Age: {props.age}</p>
</div>
);
}
ChildComponent.defaultProps = {
name: 'Anonymous',
age: 'Unknown'
};
export default ChildComponent;
In this modified ‘ChildComponent’ , if the parent component doesn’t provide ‘name’ or ‘age’ props, default values will be used instead.
Advanced usage of props with PropTypes
While React is flexible with props, it’s a good practice to define the expected types for props. This helps catch bugs early and serves as documentation for your components. React provides a built-in ‘PropTypes’ library for this purpose.
npm install prop-types
Let’s update our ‘ChildComponent’ to include PropTypes:
// ChildComponent.js
import React from 'react';
import PropTypes from 'prop-types';
function ChildComponent(props) {
return (
<div>
<p>Name: {props.name}</p>
<p>Age: {props.age}</p>
</div>
);
}
ChildComponent.propTypes = {
name: PropTypes.string.isRequired,
age: PropTypes.number.isRequired
};
export default ChildComponent;
Now, if ‘name’ or ‘age’ is not provided or doesn’t match the expected type, React will issue a warning in the console.
Real-World Example
Let’s consider a practical example where props are used extensively. Suppose we’re building a TodoList application. We have a ‘TodoList’ component that receives an array of todo items as props and renders them.
// TodoList.js
import React from 'react';
import PropTypes from 'prop-types';
import TodoItem from './TodoItem';
function TodoList({ todos }) {
return (
<div>
<h2>Todo List</h2>
<ul>
{todos.map(todo => (
<TodoItem key={todo.id} todo={todo} />
))}
</ul>
</div>
);
}
TodoList.propTypes = {
todos: PropTypes.arrayOf(
PropTypes.shape({
id: PropTypes.number.isRequired,
text: PropTypes.string.isRequired,
completed: PropTypes.bool.isRequired
})
).isRequired
};
export default TodoList;
In this example, ‘TodoList’ expects an array of todo objects as props, each having ‘id’ , ‘text’, and ‘completed’ properties. We use PropTypes to specify the expected shape of the ‘todos’ array.
Conclusion
Props are a crucial aspect of React development, allowing components to communicate and share data effectively. By mastering props, you unlock the full potential of React’s component architecture. Remember to define PropTypes for your components, use default props when necessary, and follow best practices to build robust and maintainable React applications.
Happy coding! 🚀