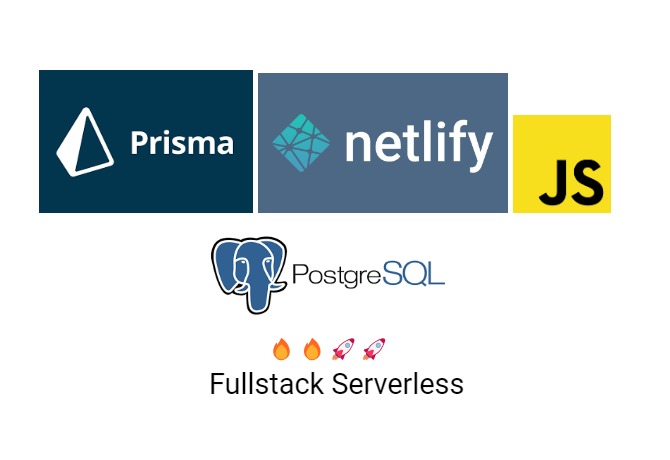
In this tutorial, we’ll walk through the process of creating a serverless Content Management System (CMS) using Netlify Functions, Prisma for database management, and PostgreSQL as the database. We’ll create models for users and posts using Prisma and support all HTTP methods for CRUD operations. Additionally, we’ll cover how to deploy the CMS to Netlify seamlessly.
Prerequisites
Before we begin, make sure you have the following prerequisites installed:
- Node.js and npm
- Netlify CLI
- Prisma CLI
- PostgreSQL
Setting Up Prisma
First,let’s set up Prisma for our project.
1.Install Prisma CLI globally:
npm install prisma -g
2.Initialize Prisma in your project directory:
prisma init
3.Choose “SQLite” for the database during intialization.
4.Define models for users and posts in ‘schema.prisma’:
model User {
id Int @id @default(autoincrement())
username String
email String @unique
posts Post[]
}
model Post {
id Int @id @default(autoincrement())
title String
content String
author User @relation(fields: [authorId], references: [id])
authorId Int
}
5.Run ‘prisma generate’ to generate Prisma Client.
Setting Up Netlify Functions
Next,let’s set up Netlify Functions.
- Install dependencies:
npm install netlify-lambda express @prisma/client
2.Create a folder named ‘functions’ at the root of your project.
3.Inside the ‘functions’ folder, create an ‘api’ folder and a ‘posts’ folder.
4.Create an ‘index.js’ file inside the ‘posts’ folder with the following content:
const express = require('express');
const { PrismaClient } = require('@prisma/client');
const prisma = new PrismaClient();
const app = express();
app.use(express.json());
app.get('/', async (req, res) => {
const posts = await prisma.post.findMany();
res.json(posts);
});
app.post('/', async (req, res) => {
const { title, content, authorId } = req.body;
const post = await prisma.post.create({
data: {
title,
content,
authorId,
},
});
res.json(post);
});
// Implement other CRUD methods similarly
module.exports = app;
Deploying to Netlify
Now, let’s deploy our serverless CMS to Netlify.
1.Initialize a Git repository in your project:
git init
2. Create a ‘.gitignore’ file and add the following entries:
node_modules/
.netlify/
3. Commit your changes:
git add .
git commit -m "Initial commit"
4. Log in to your Netlify account using the Netlify CLI:
netlify login
5. Create a new site on Netlify:
netlify init
6. Follow the prompts to link your Git repository and configure your site settings.
7. Deploy your site to Netlify:
netlify deploy --prod
8. Netlify will provide you with a URL where your CMS is deployed.
Below is the code for implementing various functions to handle users and posts CRUD operations:
// Inside the ‘users’ folder of the ‘functions’ directory
const express = require('express');
const { PrismaClient } = require('@prisma/client');
const prisma = new PrismaClient();
const app = express();
app.use(express.json());
// Get all users
app.get('/', async (req, res) => {
const users = await prisma.user.findMany();
res.json(users);
});
// Get a single user by ID
app.get('/:id', async (req, res) => {
const { id } = req.params;
const user = await prisma.user.findUnique({
where: { id: parseInt(id) }
});
if (!user) {
return res.status(404).json({ error: 'User not found' });
}
res.json(user);
});
// Create a new user
app.post('/', async (req, res) => {
const { username, email } = req.body;
const user = await prisma.user.create({
data: {
username,
email
}
});
res.json(user);
});
// Update a user by ID
app.put('/:id', async (req, res) => {
const { id } = req.params;
const { username, email } = req.body;
const updatedUser = await prisma.user.update({
where: { id: parseInt(id) },
data: {
username,
email
}
});
res.json(updatedUser);
});
// Delete a user by ID
app.delete('/:id', async (req, res) => {
const { id } = req.params;
await prisma.user.delete({
where: { id: parseInt(id) }
});
res.json({ message: 'User deleted successfully' });
});
module.exports = app;
Here’s the code for handling posts:
// Inside the ‘posts’ folder of the ‘functions’ directory
const express = require('express');
const { PrismaClient } = require('@prisma/client');
const prisma = new PrismaClient();
const app = express();
app.use(express.json());
// Get all posts
app.get('/', async (req, res) => {
const posts = await prisma.post.findMany();
res.json(posts);
});
// Get a single post by ID
app.get('/:id', async (req, res) => {
const { id } = req.params;
const post = await prisma.post.findUnique({
where: { id: parseInt(id) }
});
if (!post) {
return res.status(404).json({ error: 'Post not found' });
}
res.json(post);
});
// Create a new post
app.post('/', async (req, res) => {
const { title, content, authorId } = req.body;
const post = await prisma.post.create({
data: {
title,
content,
authorId,
},
});
res.json(post);
});
// Update a post by ID
app.put('/:id', async (req, res) => {
const { id } = req.params;
const { title, content } = req.body;
const updatedPost = await prisma.post.update({
where: { id: parseInt(id) },
data: {
title,
content,
},
});
res.json(updatedPost);
});
// Delete a post by ID
app.delete('/:id', async (req, res) => {
const { id } = req.params;
await prisma.post.delete({
where: { id: parseInt(id) },
});
res.json({ message: 'Post deleted successfully' });
});
module.exports = app;
With these functions added to your Netlify project, you’ll have endpoints for handling CRUD operations on both users and posts. This enables you to effectively manage your CMS content through HTTP requests.
Conclusion
In this tutorial, we’ve built a serverless CMS using Netlify Functions, Prisma, and PostgreSQL. We defined models for users and posts using Prisma, implemented CRUD operations with Express.js inside Netlify Functions, and deployed the CMS to Netlify. This setup provides a scalable and cost-effective solution for managing content without the need for traditional server infrastructure.
Embrace the code!