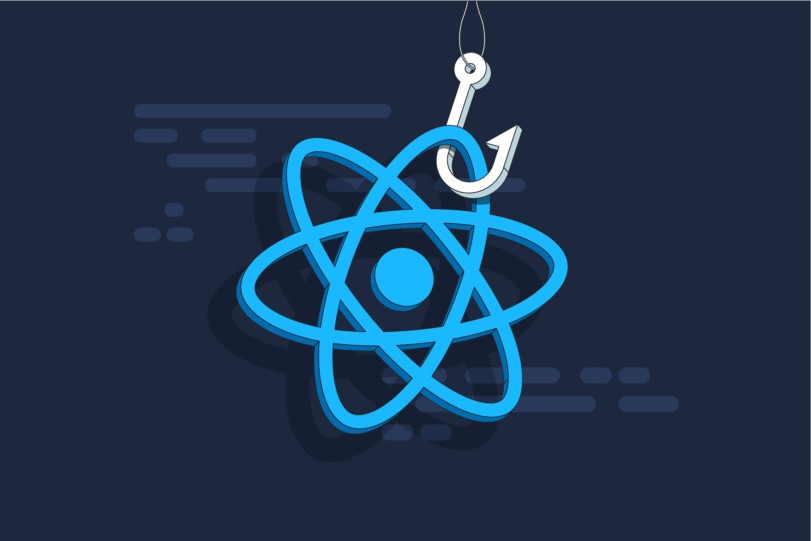
React has revolutionized the way developers build user interfaces, offering a component-based architecture that promotes reusability and simplicity. With the introduction of React Hooks, managing stateful logic in functional components has become even more intuitive and powerful. In this comprehensive guide, we will explore the benefits of adopting React Hooks for state management and delve into various hooks such as useState, useEffect, useContext, and custom hooks. By the end of this article, you will have a deep understanding of how to leverage React Hooks effectively in your projects.
1.Understanding Stateful Logic
- Stateful logic refers to the dynamic behavior of a component, where its data can change over time.
- In class components, state was managed using this.state and setState, which could lead to verbose code and complex class hierarchies.
- Functional components, on the other hand, were stateless until the introduction of React Hooks.
2.Introducing React Hooks
- React Hooks are functions that let you use state and other React features without writing a class.
- useState: The useState hook allows functional components to manage state. It returns a stateful value and a function to update it.
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
- useEffect: The useEffect hook adds the ability to perform side effects in functional components. It is similar to componentDidMount, componentDidUpdate, and componentWillUnmount combined.
import React, { useState, useEffect } from 'react';
function DataFetcher() {
const [data, setData] = useState(null);
useEffect(() => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => setData(data));
}, []); // Empty array means this effect runs once after mounting
return (
<div>
{data ? <p>Data: {data}</p> : <p>Loading...</p>}
</div>
);
}
3.Managing Complex State
- useState can manage complex state objects and arrays. You can use object destructuring to access and update individual properties.
const [user, setUser] = useState({ name: '', age: 0 });
// Updating a single property
setUser({ ...user, name: 'John' });
// Updating multiple properties
setUser({ ...user, name: 'John', age: 30 });
4.Context with useContext
- useContext hook provides a way to pass data through the component tree without having to pass props down manually at every level.
- It is especially useful for global data like themes or authentication.
import React, { useContext } from 'react';
const ThemeContext = React.createContext('light');
function ThemedButton() {
const theme = useContext(ThemeContext);
return <button style={{ background: theme }}>Click me</button>;
}
5.Custom Hooks
- Custom hooks allow you to extract component logic into reusable functions.
- They are regular JavaScript functions prefixed with ‘use’ that can call other hooks if needed.
import { useState, useEffect } from 'react';
function useDataFetcher(url) {
const [data, setData] = useState(null);
useEffect(() => {
fetch(url)
.then(response => response.json())
.then(data => setData(data));
}, [url]);
return data;
}
Best Practices for Adopting Hooks
- Embrace the Functional Paradigm: Shift your mindset from class-based lifecycle methods to functional programming concepts like pure functions and state updates.
- Leverage Custom Hooks: Extract reusable stateful logic into custom hooks for improved code organization and reusability.
- Manage Side Effects with useEffect: Utilize useEffect for side effects, ensuring proper cleanup using the cleanup function returned by the effect.
- Minimize Dependencies in useEffect: Keep dependency arrays in useEffect as concise as possible to optimize performance by only triggering re-runs when necessary.
- Use Linters and Code Formatting: Adhere to linter rules and consistent code formatting to maintain a clean and readable codebase.
Common Pitfalls
- Forgetting the dependency array in useEffect, leading to infinite loops or missed updates.
- Modifying state directly instead of using setState, which can lead to unexpected behavior.
- Incorrect usage of useContext, causing unnecessary re-renders.
Migrating from Class Components
- Converting class components to functional components with hooks is a straightforward process.
- Identify stateful logic and replace this.state and setState calls with useState.
- Replace lifecycle methods with useEffect.
- Refactor context consumers to use useContext.
Conclusion
React Hooks have simplified state management and side effects in functional components, making React development more streamlined and efficient.
By embracing hooks such as useState, useEffect, useContext, and custom hooks, developers can write cleaner, more modular code.
Understanding the principles and best practices of React Hooks is essential for mastering stateful logic in modern React applications.
React Hooks provide a powerful and elegant solution for managing stateful logic in functional components. By leveraging hooks such as useState, useEffect, useContext, and custom hooks, developers can write more concise and maintainable code. With proper understanding and adoption of React Hooks, you can take your React development skills to the next level. Happy coding!