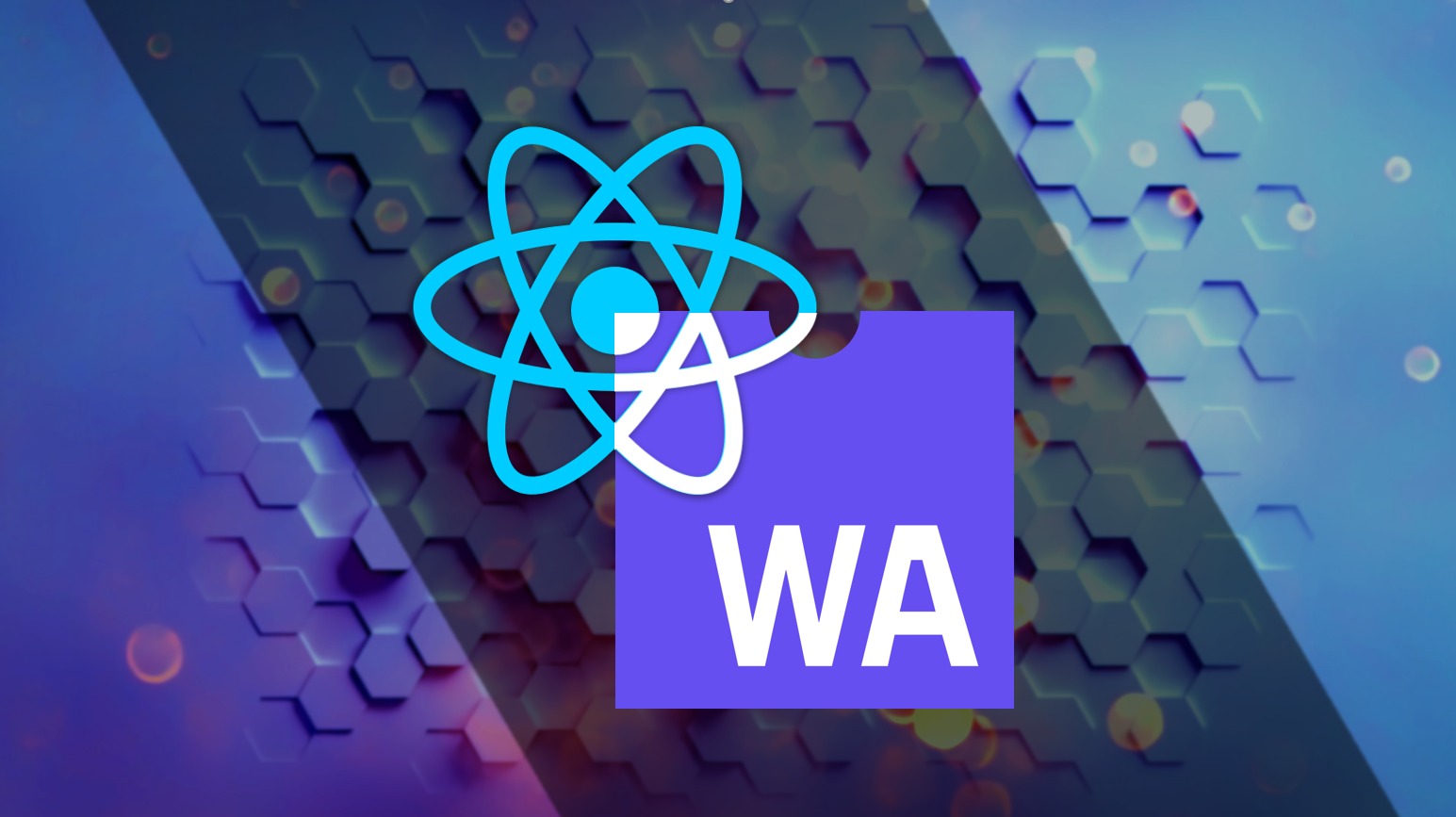
In the ever-evolving landscape of web development, two technologies have stood out for their potential to revolutionize the way we build web applications: React and WebAssembly. React, a popular JavaScript library for building user interfaces, and WebAssembly, a binary instruction format for a stack-based virtual machine, can be combined to create powerful, efficient, and highly interactive web applications. This article will delve into the basics of both technologies, their integration, and how they can be used together to enhance web development.
What is WebAssembly?
WebAssembly (often abbreviated as wasm) is a binary instruction format designed for safe and efficient execution on various platforms, including web browsers. It is intended as a portable compilation target for high-level languages like C, C++, and Rust, enabling the execution of code at near-native speed.
Key Features of WebAssembly
- Performance: WebAssembly is designed to execute at near-native speed by taking advantage of common hardware capabilities.
- Portability: WebAssembly code can run on any platform that supports it, including all major web browsers.
- Interoperability: WebAssembly is designed to work alongside JavaScript, allowing both to call each other and share functionalities.
- Security: WebAssembly runs in a safe, sandboxed environment, making it suitable for running untrusted code on the web.
Basic WebAssembly Example
Here’s a simple example of WebAssembly code written in C and compiled to WebAssembly:
C code (example.c):
#include <stdio.h>
int add(int a, int b) {
return a + b;
}
To compile this C code to WebAssembly, you can use a tool like Emscripten:
emcc example.c -o example.wasm -s EXPORTED_FUNCTIONS="['_add']" -s EXTRA_EXPORTED_RUNTIME_METHODS="['cwrap']"
Integrating React and WebAssembly
Combining React and WebAssembly allows developers to build applications that are both highly interactive and performance-efficient. WebAssembly can handle compute-intensive tasks, while React manages the user interface, providing a seamless user experience.
Setting Up the Environment
First, you’ll need to set up a basic React application. You can use Create React App to get started quickly:
npx create-react-app react-wasm-example
cd react-wasm-example
Next, you’ll need to compile your WebAssembly module. For this example, we’ll use a simple Rust function. Ensure you have Rust and the wasm-pack tool installed:
curl https://sh.rustup.rs -sSf | sh
cargo install wasm-pack
Create a new Rust project:
cargo new --lib wasm_add
cd wasm_add
Edit the ‘Cargo.toml’ file to include the ‘wasm-bindgen’ dependency:
[dependencies]
wasm-bindgen = "0.2"
Edit the ‘src/lib.rs’ file to include a simple add function
use wasm_bindgen::prelude::*;
#[wasm_bindgen]
pub fn add(a: i32, b: i32) -> i32 {
a + b
}
Compile the Rust code to WebAssembly:
wasm-pack build --target web
This command will generate a ‘pkg’ directory with the compiled WebAssembly module and JavaScript bindings.
Integrating WebAssembly with React
Now that we have our WebAssembly module, we can integrate it into our React application.
- Copy the ‘pkg ‘folder generated by wasm-pack into the ‘public’ directory of your React project.
- Load the WebAssembly module in your React component. Create a new component file ‘src/WasmComponent.js’ :
import React, { useState, useEffect } from 'react';
const WasmComponent = () => {
const [result, setResult] = useState(null);
useEffect(() => {
const loadWasm = async () => {
try {
const wasm = await import('../public/pkg/wasm_add');
setResult(wasm.add(5, 3));
} catch (err) {
console.error(`Unexpected error in loadWasm. [Message: ${err.message}]`);
}
};
loadWasm();
}, []);
return (
<div>
<h1>Wasm Add Function</h1>
{result !== null ? <p>Result: {result}</p> : <p>Loading...</p>}
</div>
);
};
export default WasmComponent;
3. Use the ‘WasmComponent’ in your main App component. Edit ‘src/App.js’ :
import React from 'react';
import WasmComponent from './WasmComponent';
import './App.css';
function App() {
return (
<div className="App">
<header className="App-header">
<WasmComponent />
</header>
</div>
);
}
export default App;
4. Run your React application:
npm start
You should see a message displaying the result of the WebAssembly add function in your React
application.
Benefits of Using React and WebAssembly Together
Performance
WebAssembly provides near-native performance for compute-intensive tasks. By offloading these tasks to WebAssembly, React applications can remain responsive and efficient.
Interoperability
WebAssembly modules can easily interact with JavaScript, allowing seamless integration with React components. This interoperability enables developers to leverage existing libraries and tools while taking advantage of WebAssembly’s performance benefits.
Improved User Experience
Combining React’s robust UI capabilities with WebAssembly’s performance allows developers to create highly interactive and performant web applications. This combination is particularly beneficial for applications that require real-time data processing, complex animations, or heavy computations.
Cross-Language Compatibility
WebAssembly allows developers to write code in multiple languages (like C, C++, and Rust) and compile it to a format that can run on the web. This flexibility enables the use of existing codebases and libraries, reducing development time and effort.
Advanced Integration Techniques
Handling Complex Data Structures
While simple data types like integers and floats can be easily passed between JavaScript and WebAssembly, handling complex data structures requires additional work. The ‘wasm-bindgen’ library provides tools to handle this complexity.
For example, to pass an array from JavaScript to WebAssembly:
Rust code (‘ src/lib.rs ‘) :
use wasm_bindgen::prelude::*;
#[wasm_bindgen]
pub fn sum_array(arr: &[i32]) -> i32 {
arr.iter().sum()
}
JavaScript code (src/WasmComponent.js):
import React, { useState, useEffect } from 'react';
const WasmComponent = () => {
const [result, setResult] = useState(null);
useEffect(() => {
const loadWasm = async () => {
try {
const wasm = await import('../public/pkg/wasm_add');
const arr = new Int32Array([1, 2, 3, 4, 5]);
setResult(wasm.sum_array(arr));
} catch (err) {
console.error(`Unexpected error in loadWasm. [Message: ${err.message}]`);
}
};
loadWasm();
}, []);
return (
<div>
<h1>Wasm Sum Array Function</h1>
{result !== null ? <p>Result: {result}</p> : <p>Loading...</p>}
</div>
);
};
export default WasmComponent;
Error Handling and Debugging
Handling errors and debugging WebAssembly code can be challenging due to its low-level nature. Tools like ‘wasm-bindgen’ and ‘console_error_panic_hook’ can help by providing better error messages and stack traces.
To use ‘console_error_panic_hook’ , add it to your Rust project:
[dependencies]
console_error_panic_hook = "0.1"
wasm-bindgen = "0.2"
Update your ‘src/lib.rs’ file:
use wasm_bindgen::prelude::*;
use std::panic;
#[wasm_bindgen(start)]
pub fn main() {
panic::set_hook(Box::new(console_error_panic_hook::hook));
}
#[wasm_bindgen]
pub fn add(a: i32, b: i32) -> i32 {
a + b
}
This setup ensures that any panic in your WebAssembly code will print a detailed error message
in the browser console.
Using Third-Party Libraries
WebAssembly can be used with a variety of third-party libraries to enhance functionality. For instance, you can use libraries like ‘wee_alloc’ to reduce the size of your WebAssembly binary or ‘js-sys’ and ‘web-sys’ to interact with JavaScript and the Web API.
Conclusion
React and WebAssembly are powerful technologies that, when used together, can significantly enhance the performance and capabilities of web applications. React’s component-based architecture and efficient rendering system, combined with WebAssembly’s near-native execution speed, allow developers to build modern, high-performance web applications.
By following the steps outlined in this article, you can start integrating WebAssembly into your React projects, leveraging the strengths of both technologies to create exceptional web applications. As the web continues to evolve, the combination of React and WebAssembly will undoubtedly play a crucial role in shaping the future of web development.