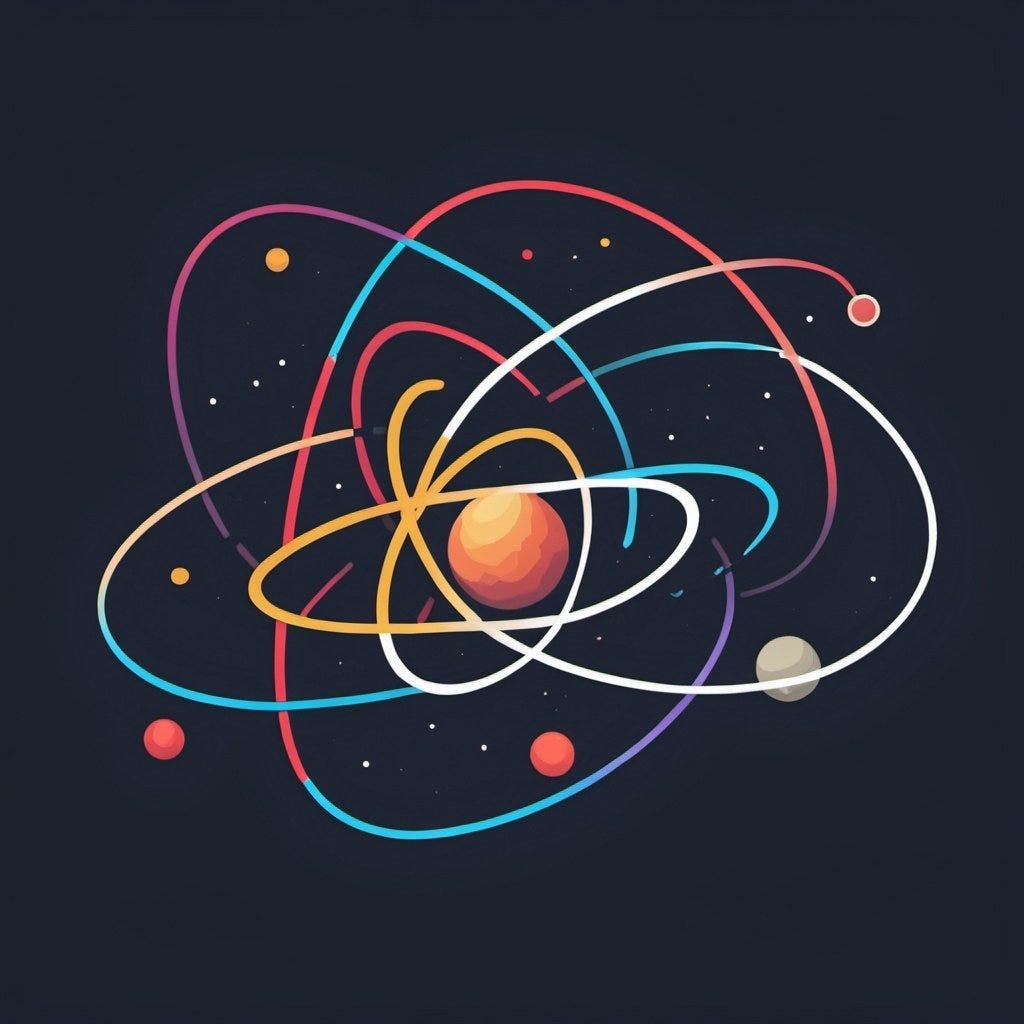
In today’s rapidly evolving digital landscape, the need for robust, efficient, and user-friendly web applications has never been greater. Progressive Web Apps (PWAs) have emerged as a revolutionary solution, combining the best of web and mobile apps to deliver seamless user experiences. Simultaneously, frameworks like Astro have been developed to simplify the creation of these advanced applications. This article delves into the world of PWAs and Astro, exploring their benefits, functionalities, and how to leverage them to build cutting-edge web applications.
Table of Contents
- Introduction to Progressive Web Apps
- Introduction to Astro
- Building a PWA with Astro
- Code Examples
- Conclusion
Introduction to Progressive Web Apps
Progressive Web Apps (PWAs) are web applications that use modern web capabilities to deliver an app-like experience to users. They are built using standard web technologies such as HTML, CSS, and JavaScript but offer functionalities traditionally associated with native apps, like offline capabilities, push notifications, and access to device hardware.
Introduction to Astro
Astro is a modern framework for building fast, optimized websites. Unlike traditional JavaScript frameworks, Astro focuses on delivering the best performance by minimizing client-side JavaScript and emphasizing static site generation. This approach makes it an ideal choice for building PWAs.
Key Features of Astro
- Zero JavaScript Runtime: Only loads JavaScript when necessary.
- Islands Architecture: Splits the page into smaller, isolated components that can be loaded independently.
- SEO-Friendly: Static site generation ensures content is easily indexable.
- Easy to Learn: Familiar syntax for developers already versed in HTML, CSS, and JavaScript.
- Highly Performant: Prioritizes performance by default.
Building a PWA with Astro
Creating a PWA with Astro involves a few key steps:
- Setting Up the Astro Project: Install Astro and create a new project.
- Creating the PWA Manifest: Define the app’s metadata and how it should appear to users.
- Service Workers: Implement a service worker to handle offline functionality and caching.
- Enhancing the App: Add features like push notifications and responsive design.
Step-by-Step Guide
Step 1: Setting Up the Astro Project
First, you need to set up your Astro project. Ensure you have Node.js installed, then run the following commands:
npm init astro
cd my-astro-pwa
npm install
This initializes a new Astro project in a directory called ‘my-astro-pwa’.
Step 2: Creating the PWA Manifest
Create a ‘manifest.json’ file in the ‘public’ directory:
{
"name": "My Astro PWA",
"short_name": "AstroPWA",
"description": "An example Progressive Web App built with Astro",
"start_url": "/",
"display": "standalone",
"background_color": "#ffffff",
"theme_color": "#333333",
"icons": [
{
"src": "/icons/icon-192x192.png",
"sizes": "192x192",
"type": "image/png"
},
{
"src": "/icons/icon-512x512.png",
"sizes": "512x512",
"type": "image/png"
}
]
}
This manifest file describes your PWA and how it should behave when installed on a device.
Step 3: Implementing Service Workers
Service workers are scripts that run in the background and handle caching and offline functionality. Create a ‘sw.js’ file in the ‘public’ directory:
const CACHE_NAME = 'astro-pwa-cache';
const urlsToCache = [
'/',
'/index.html',
'/styles.css',
'/scripts.js'
];
// Install a service worker
self.addEventListener('install', event => {
event.waitUntil(
caches.open(CACHE_NAME)
.then(cache => {
return cache.addAll(urlsToCache);
})
);
});
// Cache and return requests
self.addEventListener('fetch', event => {
event.respondWith(
caches.match(event.request)
.then(response => {
return response || fetch(event.request);
})
);
});
// Update a service worker
self.addEventListener('activate', event => {
const cacheWhitelist = [CACHE_NAME];
event.waitUntil(
caches.keys().then(cacheNames => {
return Promise.all(
cacheNames.map(cacheName => {
if (cacheWhitelist.indexOf(cacheName) === -1) {
return caches.delete(cacheName);
}
})
);
})
);
});
Register the service worker in your ‘index.html’ file:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="manifest" href="/manifest.json">
<title>My Astro PWA</title>
</head>
<body>
<h1>Welcome to My Astro PWA</h1>
<script>
if ('serviceWorker' in navigator) {
window.addEventListener('load', () => {
navigator.serviceWorker.register('/sw.js')
.then(registration => {
console.log('ServiceWorker registration successful with scope: ', registration.scope);
}, error => {
console.log('ServiceWorker registration failed: ', error);
});
});
}
</script>
</body>
</html>
Step 4: Enhancing the App
Enhance your PWA by adding responsive design and additional features. For example, you can use CSS to ensure your app looks great on all devices:
/* styles.css */
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
background-color: #f0f0f0;
color: #333;
}
h1 {
text-align: center;
margin-top: 20px;
}
.container {
max-width: 1200px;
margin: auto;
padding: 20px;
}
@media (max-width: 600px) {
.container {
padding: 10px;
}
}
Example Astro Component
Here’s an example of an Astro component:
// src/components/Header.astro
const siteTitle = 'My Astro PWA';
---
<header>
<h1>{siteTitle}</h1>
<nav>
<ul>
<li><a href="/">Home</a></li>
<li><a href="/about">About</a></li>
</ul>
</nav>
</header>
<style>
header {
background-color: #333;
color: #fff;
padding: 10px 0;
}
nav ul {
list-style: none;
padding: 0;
}
nav li {
display: inline;
margin-right: 10px;
}
nav a {
color: #fff;
text-decoration: none;
}
</style>
Conclusion
Progressive Web Apps and Astro represent a powerful combination for building modern, performant web applications. PWAs offer the benefits of native apps while retaining the flexibility and reach of the web. Astro, with its emphasis on performance and simplicity, provides an excellent framework for creating these advanced applications.
By following the steps outlined in this article, you can create your own PWA using Astro, leveraging the strengths of both technologies to deliver an outstanding user experience. Whether you’re a seasoned developer or just getting started, the combination of PWAs and Astro will equip you with the tools you need to build the future of the web. Happy coding!