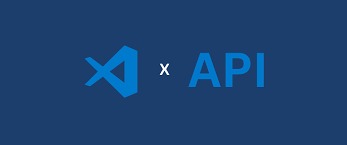
Creating a Visual Studio Code (VS Code) extension can be a fun and rewarding experience, especially when you see your tool being used by developers around the world. In this article, we’ll walk through building a VS Code extension that fetches and displays content from an API. We’ll break down the process into simple steps and include code snippets to guide you through the development. Let’s dive in! 🌊
Table of Contents
- Introduction
- Prerequisites
- Setting Up the Project
- Creating the Extension
- Fetching Data from an API
- Displaying Data in VS Code
- Running and Debugging the Extension
- Publishing the Extension
- Conclusion
Introduction
Extensions are a powerful way to enhance the capabilities of VS Code. By fetching and displaying data from an API, you can integrate external services directly into your development environment. This guide will show you how to create a VS Code extension that retrieves data from a public API and displays it in a user-friendly way. 🚀
Getting Started 🏁
Before we start, make sure you have the following prerequisites:
- Node.js installed on your machine
- Visual Studio Code installed
- Basic understanding of JavaScript/TypeScript
Step 1: Setting Up Your Development Environment 🛠️
First, we’ll set up our development environment. We’ll use the Yeoman generator for VS Code extensions to scaffold our project.
1.Install Yeoman and the VS Code Extension Generator:
npm install -g yo generator-code
2.Create a new extension:
yo code
Follow the prompts to set up your project. For example:
- What type of extension do you want to create? New Extension (TypeScript)
- What’s the name of your extension? api-fetcher
- What’s the identifier of your extension? api-fetcher
- What’s the description of your extension? A VS Code extension to fetch and display content from an API.
- Initialize a git repository? Yes
- Which package manager to use? npm
Yeoman will generate the project structure and install the necessary dependencies. You should see a new directory with your extension’s name.
Creating the Extension
Let’s dive into the code! Open the generated project in VS Code.
1.Open src/extension.ts: This file contains the main logic for your extension.
2’Add the following imports at the top of the file:
import * as vscode from 'vscode';
import fetch from 'node-fetch';
3.Modify the ‘activate’ function to register a new command:
export function activate(context: vscode.ExtensionContext) {
let disposable = vscode.commands.registerCommand('api-fetcher.fetchData', async () => {
const panel = vscode.window.createWebviewPanel(
'apiFetcher',
'API Data',
vscode.ViewColumn.One,
{}
);
const data = await fetchData();
panel.webview.html = getWebviewContent(data);
});
context.subscriptions.push(disposable);
}
4.Create a new function to fetch data from the API:
async function fetchData(): Promise<any> {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
return data;
}
5.Create a function to generate HTML content for the webview:
function getWebviewContent(data: any): string {
return `
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>API Data</title>
</head>
<body>
<h1>API Data</h1>
<pre>${JSON.stringify(data, null, 2)}</pre>
</body>
</html>
`;
}
Fetching Data from an API
In this example, we’re using a placeholder API endpoint (‘https://api.example.com/data’). You can replace it with any API you like. The ‘fetchData’ function uses the ‘node-fetch’ package to make HTTP requests. If your API requires authentication, you’ll need to add the necessary headers to the request.
Displaying Data in VS Code
The fetched data is displayed in a webview panel. Webviews are custom-styled HTML-based views that you can embed in VS Code. The ‘getWebviewContent’ function generates the HTML content, which is then set as the webview’s content.
Running and Debugging the Extension
- Open the’ Run and Debug’ view in VS Code.
- Click on’ Run Extension’: This will open a new VS Code window with your extension loaded.
- Press ‘Ctrl+Shift+P’ and run the ‘API Fetcher: Fetch Data’ command: You should see a new webview panel with the fetched data.
Publishing the Extension
Once you’re happy with your extension, you can publish it to the Visual Studio Code Marketplace.
- Create a publisher: If you don’t have one, create a publisher account on the Visual Studio Code Marketplace.
- Login to vsce: The vsce tool is used to package and publish extensions.
npm install -g vsce
vsce login <publisher-name>
3.Package the extension:
vsce package
4.Publish the extension:
vsce publish
Conclusion
Congratulations! You’ve built a VS Code extension that fetches and displays content from an API. This is just the beginning – you can extend this basic functionality to include more complex interactions, error handling, and even user inputs.
Creating VS Code extensions can greatly enhance your development workflow, and integrating API data directly into your editor can provide real-time insights and data-driven decisions. Happy coding! 🎉