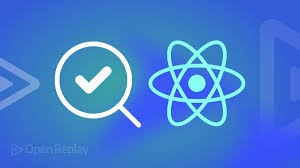
Understanding Mock Objects in React
1. Introduction
- What are Mock Objects?
- Why Use Mocks in Testing?
- Importance of Mocking in React
2. Understanding Mock Objects in React
- What is a Mock Object in React?
- Common Use Cases for Mocks in React Testing
3. Setting Up the Environment
- Tools and Libraries Required
- Installing Jest and React Testing Library
- Setting Up a Basic React Project for Testing
4. Creating and Using Mock Objects
- Mocking Functions
- Example: Mocking a Simple Function
- Example: Mocking a Function with External API Calls
- Mocking Components
- Example: Mocking Child Components
- Example: Mocking Third-Party Components
- Mocking Modules
- Example: Mocking an Entire Module
- Example: Mocking Specific Exports from a Module
5. Advanced Mocking Techniques
- Using Jest Mocks for Advanced Scenarios
- Mocking Network Requests with Axios
- Mocking Time-Dependent Code
6. Real-World Examples
- Testing a React Component with Mocks
- Mocking Redux in React
- Mocking Context API
7. Best Practices for Mocking in React
- When to Mock and When Not To
- Keeping Tests Maintainable
- Ensuring Realistic Mock Data
8. Conclusion
- Recap of Key Points
- Final Thoughts on Mocking in React
Introduction
What are Mock Objects?
Mock objects are simulated objects that mimic the behavior of real objects in controlled ways. They are used in software testing to isolate the component being tested from its dependencies, allowing for more focused and reliable tests. In the context of React, mock objects can be used to simulate everything from simple functions to entire components or modules.
Why Use Mocks in Testing?
Using mocks allows developers to:
- Isolate Units of Code: Focus on testing specific components or functions without worrying about the complexities of their dependencies.
- Speed Up Tests: Mocks can replace time-consuming operations like network requests, making tests run faster.
- Control Test Scenarios: By mocking objects, you can simulate various edge cases and error conditions that might be difficult to reproduce with real objects.
Importance of Mocking in React
React is a component-based library that often relies on external APIs, libraries, and internal states. When testing React applications, it’s crucial to ensure that each component works correctly in isolation. Mocking allows you to simulate props, state, API calls, and other dependencies, making it easier to test components thoroughly.
Understanding Mock Objects in React
What is a Mock Object in React?
In React, a mock object can be a function, component, or module that is replaced with a simulated version. This simulated version behaves as expected in a controlled manner, allowing the test to focus on the functionality of the component under test.
For example, consider a React component that fetches data from an API:
import React, { useEffect, useState } from 'react';
const DataFetcher = ({ api }) => {
const [data, setData] = useState(null);
useEffect(() => {
api.fetchData().then(response => setData(response));
}, [api]);
if (!data) {
return <div>Loading...</div>;
}
return <div>Data: {data}</div>;
};
export default DataFetcher;
To test this component, you don’t want to make actual API calls, so you mock the ‘api.fetchData’ function.
Common Use Cases for Mocks in React Testing
- Mocking API Calls: Replace network requests with mock functions to simulate various responses.
- Mocking Child Components: Simplify tests by replacing complex child components with mocks.
- Mocking Modules: Replace entire modules, like utility libraries, with mock versions.
- Mocking Time-Based Functions: Control time-dependent logic using mocks for functions like ‘setTimeout’ or ‘Date’.
Setting Up the Environment
Tools and Libraries Required
To work with mock objects in React, you’ll need the following tools and libraries:
- React: The core library for building user interfaces.
- Jest: A popular testing framework that comes with built-in support for mocking.
- React Testing Library: A library for testing React components in a way that mimics how users interact with your app.
Installing Jest and React Testing Library
To get started, create a new React project and install the necessary dependencies:
npx create-react-app react-mock-demo
cd react-mock-demo
npm install --save-dev jest @testing-library/react @testing-library/jest-dom
Setting Up a Basic React Project for Testing
Once you’ve installed the dependencies, create a simple React component that you’ll be testing:
// src/components/Greeting.js
import React from 'react';
const Greeting = ({ name }) => {
return <div>Hello, {name}!</div>;
};
export default Greeting;
Now, create a test file for this component:
// src/components/Greeting.test.js
import React from 'react';
import { render } from '@testing-library/react';
import Greeting from './Greeting';
test('renders greeting with name', () => {
const { getByText } = render(<Greeting name="John" />);
expect(getByText('Hello, John!')).toBeInTheDocument();
});
Run the test using ‘npm test’, and it should pass.
Creating and Using Mock Objects
Mocking Functions
Example: Mocking a Simple Function
Let’s start by mocking a simple function. Consider a component that calls a function to get a message:
import React from 'react';
const Message = ({ getMessage }) => {
return <div>{getMessage()}</div>;
};
export default Message;
To test this component, you can mock the ‘getMessage’ function:
import React from 'react';
import { render } from '@testing-library/react';
import Message from './Message';
test('renders message', () => {
const mockGetMessage = jest.fn().mockReturnValue('Hello, World!');
const { getByText } = render(<Message getMessage={mockGetMessage} />);
expect(getByText('Hello, World!')).toBeInTheDocument();
});
Example: Mocking a Function with External API Calls
Now, consider a function that makes an API call:
import axios from 'axios';
export const fetchData = async () => {
const response = await axios.get('/api/data');
return response.data;
};
To mock this function in a test:
import axios from 'axios';
import { fetchData } from './api';
jest.mock('axios');
test('fetches data from API', async () => {
axios.get.mockResolvedValue({ data: 'mocked data' });
const data = await fetchData();
expect(data).toBe('mocked data');
});
Mocking Components
Example: Mocking Child Components
Sometimes, you want to mock child components to simplify testing:
import React from 'react';
const Parent = () => {
return (
<div>
<Child />
</div>
);
};
const Child = () => {
return <div>Child Component</div>;
};
export default Parent;
In the test:
import React from 'react';
import { render } from '@testing-library/react';
import Parent from './Parent';
jest.mock('./Child', () => () => <div>Mocked Child Component</div>);
test('renders mocked child component', () => {
const { getByText } = render(<Parent />);
expect(getByText('Mocked Child Component')).toBeInTheDocument();
});
Example: Mocking Third-Party Components
You can also mock third-party components:
jest.mock('react-router-dom', () => ({
Link: () => <div>Mocked Link</div>,
}));
Mocking Modules
Example: Mocking an Entire Module
To mock an entire module:
jest.mock('./api');
import * as api from './api';
test('mocks entire module', () => {
api.fetchData.mockResolvedValue('mocked data');
// Your test code
});
Example: Mocking Specific Exports from a Module
To mock specific exports:
import { fetchData } from './api';
jest.mock('./api', () => ({
fetchData: jest.fn(),
}));
test('mocks specific export', () => {
fetchData.mockResolvedValue('mocked data');
// Your test code
});
5. Advanced Mocking Techniques
Using Jest Mocks for Advanced Scenarios
Jest allows for more complex mocking scenarios, like mocking multiple calls with different return values:
const mockFunction = jest.fn()
.mockReturnValueOnce('first call')
.mockReturnValueOnce('second call');
expect(mockFunction()).toBe('first call');
expect(mockFunction()).toBe('second call');
Mocking Network Requests with Axios
To mock network requests, use ‘axios’ mocks:
jest.mock('axios');
axios.get.mockImplementation(() => Promise.resolve({ data: 'mocked data' }));
// Your test code
Mocking Time-Dependent Code
To mock time-dependent functions like ‘setTimeout’:
jest.useFakeTimers();
test('mocks setTimeout', () => {
const mockCallback = jest.fn();
setTimeout(mockCallback, 1000);
jest.advanceTimersByTime(1000);
expect(mockCallback).toHaveBeenCalledTimes(1);
});
6. Real-World Examples
Testing a React Component with Mocks
Consider a React component that fetches data and displays it:
import React, { useEffect, useState } from 'react';
import { fetchData } from './api';
const DataComponent = () => {
const [data, setData] = useState(null);
useEffect(() => {
fetchData().then(response => setData(response));
}, []);
return <div>{data}</div>;
};
export default DataComponent;
To test this:
import React from 'react';
import { render, waitFor } from '@testing-library/react';
import DataComponent from './DataComponent';
import { fetchData } from './api';
jest.mock('./api');
test('fetches and displays data', async () => {
fetchData.mockResolvedValue('mocked data');
const { getByText } = render(<DataComponent />);
await waitFor(() => expect(getByText('mocked data')).toBeInTheDocument());
});
Mocking Redux in React
If your React component uses Redux:
import { Provider } from 'react-redux';
import { createStore } from 'redux';
const mockStore = createStore(() => ({ key: 'value' }));
render(
<Provider store={mockStore}>
<YourComponent />
</Provider>
);
Mocking Context API
To mock the Context API:
import { MyContext } from './MyContext';
jest.mock('./MyContext', () => ({
MyContext: {
Consumer: ({ children }) => children('mocked value'),
},
}));
// Your test code
Best Practices for Mocking in React
When to Mock and When Not To
- Mock Sparingly: Only mock dependencies that are not the focus of the test.
- Use Real Objects When Possible: Prefer real objects over mocks to ensure your tests are as realistic as possible.
Keeping Tests Maintainable
- Use Descriptive Names: Name your mock objects and functions clearly to reflect their purpose.
- Clean Up Mocks: Use ‘jest.clearAllMocks()’ to reset mocks between tests to avoid state leakage.
Ensuring Realistic Mock Data
- Use Realistic Data: Your mocks should return data that closely resembles what would be returned in production.
- Simulate Edge Cases: Use mocks to test edge cases and ensure your components handle them gracefully.
Conclusion
Mock objects are essential tools for writing reliable and maintainable tests in React applications. By simulating dependencies such as functions, components, and external modules, you can focus on testing the core logic of your components without worrying about external factors. This approach not only improves the accuracy of your tests but also speeds up the testing process, making it easier to identify and fix issues.
Effective mocking is about balance. Over-mocking can lead to tests that are disconnected from reality, while under-mocking can make tests brittle and slow. The key is to mock what you need to isolate, while still ensuring that your tests reflect how your components will behave in a real environment.
As you continue to write and refine your tests, remember to use realistic mock data, simulate various scenarios—including edge cases—and keep your tests clean and maintainable. By following the best practices and techniques outlined in this article, you’ll be well-equipped to create robust tests that ensure your React applications are reliable, performant, and ready for production.
In summary, mastering the art of mocking in React will make your tests more focused, your development process more efficient, and your applications more resilient. With the right approach, you can confidently deliver high-quality software that meets both your technical requirements and your users’ needs.