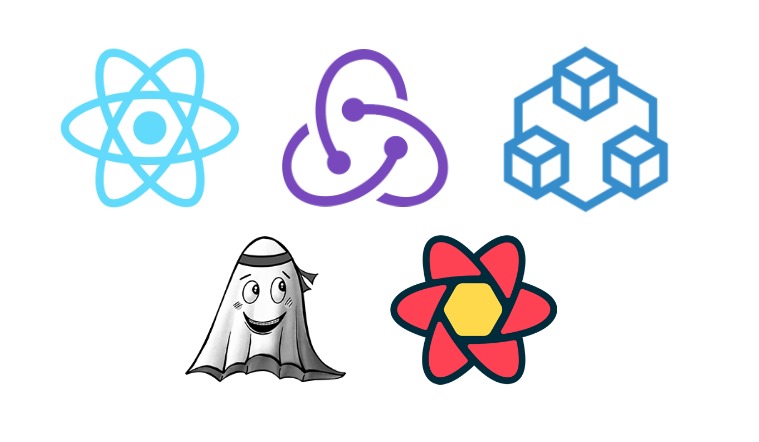
Introduction:
State management is a crucial aspect of building scalable and maintainable React applications, especially as they grow in complexity. Two popular libraries, Redux and MobX, have emerged as go-to solutions for managing state in React applications. In this article, we will delve into the intricacies of both Redux and MobX, compare their approaches, and provide insights to help you make an informed decision for your project.
Understanding Redux:
Redux is a predictable state container for JavaScript applications. At its core, Redux maintains a single immutable state tree that serves as the source of truth for an entire application. Actions are dispatched to describe state changes, and pure functions called reducers handle these actions to produce a new state.
Redux Code Example:
Letโs take a look at a simple Redux setup to get a feel for its architecture.
// actions.js
export const increment = () => ({ type: 'INCREMENT' });
export const decrement = () => ({ type: 'DECREMENT' });
// reducers.js
const counterReducer = (state = 0, action) => {
switch (action.type) {
case 'INCREMENT':
return state + 1;
case 'DECREMENT':
return state - 1;
default:
return state;
}
};
export default counterReducer;
//store.js
import { createStore } from 'redux';
import counterReducer from './reducers';
const store = createStore(counterReducer);
export default store;
Understanding MobX:
MobX, short for “Observables,” takes a different approach. It allows you to create an observable state and automatically tracks and updates components that depend on that state. In MobX, there’s no need for actions or reducers; instead, you directly modify the state, and MobX takes care of updating the components.
MobX Code Example:
Hereโs a simple MobX setup for a counter application:
import { makeObservable, observable, action } from 'mobx';
class CounterStore {
count = 0;
constructor() {
makeObservable(this, {
count: observable,
increment: action,
decrement: action,
});
}
increment() {
this.count += 1;
}
decrement() {
this.count -= 1;
}
}
const counterStore = new CounterStore();
export default counterStore;
Comparing Redux and MobX:
1.Complexity and Boilerplate:
. Redux typically requires more boilerplate code due to actions, reducers, and the store setup.
. MobX simplifies state management by eliminating the need for actions and reducers, resulting in less boilerplate.
2.Flexibility:
. Redux’s strict structure provides a clear separation of concerns, making it suitable for large-scale applications with complex state logic.
. MobX is more flexible and forgiving, allowing for a more straightforward development process but may lead to less structure in larger applications.
3.Performance:
.Redux’s immutability and pure functions can contribute to performance optimizations, especially with the use of middleware like Reselect.
. MobX’s automatic reactivity can lead to highly optimized re-rendering, but it requires careful management of observable data to avoid unnecessary updates.Choosing the Right One for Your Project:
1.Project Size:
For smaller projects with simpler state management needs, MobX’s simplicity may be more appealing.
.For larger projects with complex state interactions, Redux’s structured approach provides better maintainability.
2.Developer Experience:
. Consider the preferences and experience of your development team. Some developers may prefer the explicit structure of Redux, while others may appreciate MobX’s flexibility.
3.Learning Curve:.Redux has a steeper learning curve due to its concepts of actions,reducers,and middleware.
.MobXโs learning curve is generally considered more approachable,making it suitable for developers new to state management.
Conclusion:
In conclusion, both Redux and MobX offer effective solutions for state management in React applications. The choice between them ultimately depends on the specific requirements and preferences of your project and development team. Redux provides a structured and scalable approach, while MobX offers simplicity and flexibility. By understanding the strengths and trade-offs of each library, you can make an informed decision to ensure the success of your React application.