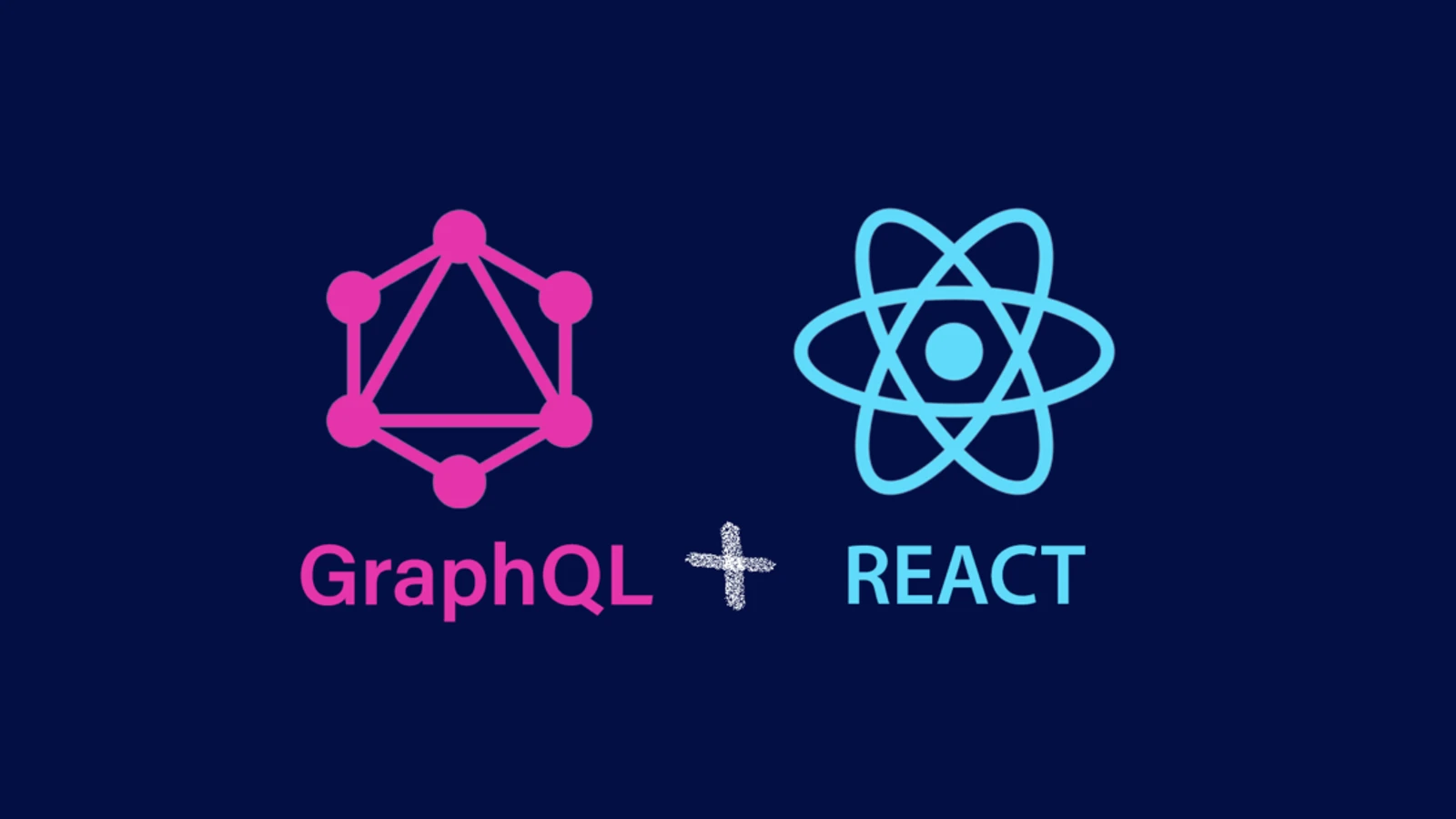
In the realm of web development, efficiency is paramount. Users expect seamless experiences, and developers strive to meet these expectations through innovative technologies and methodologies. Among these, GraphQL and React stand out as a dynamic duo, offering a powerful combination for efficient data fetching and management in modern web applications.
It provides a flexible and efficient alternative to traditional REST APIs by enabling clients to request exactly the data they need, in a single request, and in a structured format defined by the client. This eliminates issues such as over-fetching or under-fetching of data, common in RESTful architectures.
React, on the other hand, is a JavaScript library for building user interfaces, It allows developers to create reusable UI components that efficiently update in response to changes in data, making it ideal for building complex and interactive web applications.
When combined, GraphQL and React complement each other perfectly, offering a seamless development experience and optimized performance. Let’s explore how these two technologies work together to achieve efficient data fetching and management:
Declarative Data Fetching with GraphQL:
GraphQL enables developers to describe their data requirements using declarative syntax. Instead of relying on fixed endpoints and predefined data structures, clients can specify exactly what data they need using a GraphQL query. This flexibility allows for efficient data fetching, as clients can fetch only the data they require for a particular view or component.
// Example GraphQL Query
query {
user(id: "123") {
name
email
posts {
title
content
}
}
}
Efficient Component Rendering with React:
React’s component-based architecture allows developers to create encapsulated UI components that manage their state and render efficiently. When combined with GraphQL, React components can fetch and display data dynamically, updating only the relevant parts of the UI when the underlying data changes. This results in a more responsive user experience and optimized performance.
// Example React Component using GraphQL with Apollo Client
import { useQuery, gql } from '@apollo/client';
const GET_USER = gql`
query GetUser($userId: ID!) {
user(id: $userId) {
name
email
posts {
title
content
}
}
}
`;
const UserDetails = ({ userId }) => {
const { loading, error, data } = useQuery(GET_USER, {
variables: { userId },
});
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
return (
<div>
<h1>{data.user.name}</h1>
<p>Email: {data.user.email}</p>
<h2>Posts</h2>
<ul>
{data.user.posts.map(post => (
<li key={post.title}>
<h3>{post.title}</h3>
<p>{post.content}</p>
</li>
))}
</ul>
</div>
);
};
export default UserDetails;
Optimized Network Requests
GraphQL’s ability to fetch multiple resources in a single request helps minimize network overhead and reduce latency. By consolidating data fetching into a single request, GraphQL reduces the number of round trips between the client and server, resulting in faster data retrieval and improved performance. This is particularly beneficial for mobile devices and low-bandwidth environments where network latency is a concern.
Normalized Data Store with Apollo Client
Apollo Client is a popular GraphQL client for React applications that provides a normalized cache for storing and managing data fetched from a GraphQL API. By normalizing data, Apollo Client ensures that each piece of data is stored only once in the cache, reducing memory consumption and improving performance. This also facilitates efficient data updates and ensures consistency across the application.
Real-time Data Updates with Subscriptions
GraphQL supports real-time data updates through subscriptions, allowing clients to subscribe to changes in data and receive updates in real-time. When combined with React, subscriptions enable developers to build interactive and collaborative applications that respond to changes instantly, without the need for manual polling or refreshing.
Developer Experience and Tooling
Both GraphQL and React have rich ecosystems of tools and libraries that enhance the developer experience and streamline development workflows. Tools like GraphQL Playground and GraphiQL provide graphical interfaces for exploring and testing GraphQL APIs, while React Developer Tools offer powerful debugging and profiling capabilities for React applications. Additionally, frameworks like Next.js and Gatsby integrate seamlessly with GraphQL and React, providing a solid foundation for building production-ready web applications.
Conclusion
GraphQL and React form a dynamic duo for efficient data fetching and management in modern web applications. By leveraging GraphQL’s declarative data fetching capabilities and React’s component-based architecture, developers can build highly responsive and performant user interfaces that adapt seamlessly to changes in data. With optimized network requests, normalized data storage, and real-time updates, GraphQL and React empower developers to create rich and engaging user experiences that meet the demands of today’s web applications.
This synergy between GraphQL and React not only improves developer productivity but also enhances the overall user experience, making it a winning combination for building the next generation of web applications. As the web continues to evolve, GraphQL and React will undoubtedly remain at the forefront of innovation, driving the future of web development forward.