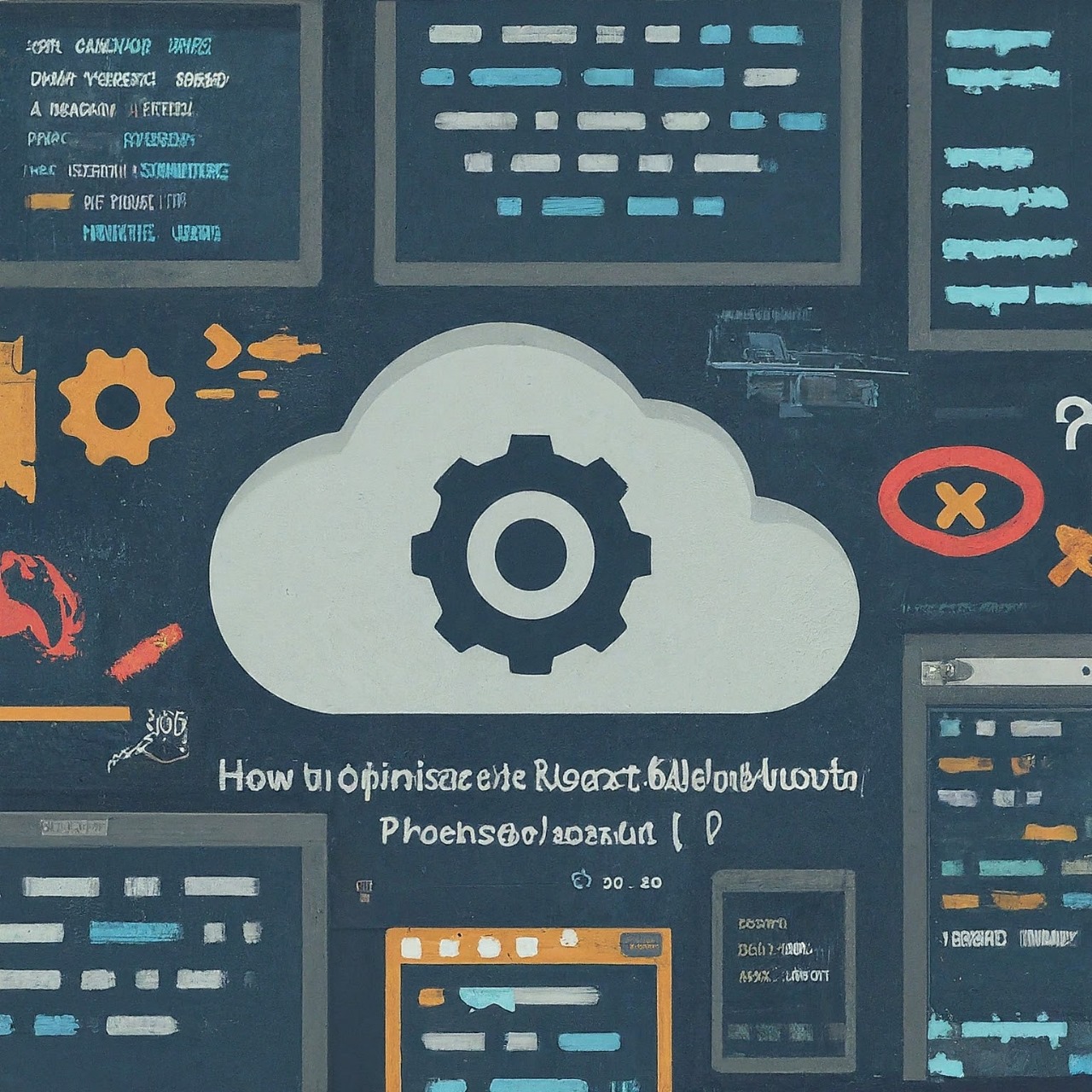
React has become the go-to JavaScript library for building user interfaces, from small prototypes to large-scale production applications. As your React project evolves from a simple prototype to a full-fledged production application, optimizing your build workflow becomes crucial. In this article, we’ll explore various techniques and best practices to streamline your React build process, from development to deployment.
Setting Up Your Development Environment
Before diving into optimizing the build workflow, let’s ensure we have a solid development environment in place. Here are the essential tools and configurations you’ll need:
1. Node.js and npm/yarn
Ensure you have Node.js installed on your system, along with npm or yarn for managing dependencies and running scripts.
2. Create React App
Utilize Create React App for bootstrapping your React project. It sets up your development environment with all the necessary configurations, such as webpack and Babel, out of the box.
npx create-react-app my-app
cd my-app
3. ESLint and Prettier
Integrate ESLint and Prettier to maintain code quality and formatting consistency across your project.
npm install eslint prettier eslint-plugin-react eslint-plugin-react-hooks eslint-config-prettier eslint-plugin-prettier --save-dev
4. Git Hooks
Implement Git hooks to enforce code quality checks and tests before committing changes.
npm install husky lint-staged --save-dev
Now that we have our development environment set up let’s move on to optimizing our build workflow.
Streamlining Development Workflow
During the development phase, developers aim for a fast feedback loop to iterate quickly. Here’s how you can optimize your development workflow:
1. Hot Reloading
Take advantage of React’s hot reloading feature to see changes instantly without a full page reload
// webpack.config.js
module.exports = {
// ...
devServer: {
hot: true,
},
};
2. Source Maps
Enable source maps to debug your code efficiently in the browser’s developer tools.
// webpack.config.js
module.exports = {
// ...
devtool: 'inline-source-map',
};
3. Environment Variables
Manage environment-specific configurations using .env files.
REACT_APP_API_URL=https://api.example.com
// src/api.js
const apiUrl = process.env.REACT_APP_API_URL;
Optimizing Production Build
Once your application is ready for deployment, optimizing the production build becomes crucial for performance and user experience. Here’s how you can achieve that:
1. Minification and Compression
Minify and compress your JavaScript, CSS, and HTML files to reduce their size and improve load times.
// webpack.config.js
const TerserPlugin = require('terser-webpack-plugin');
const CompressionPlugin = require('compression-webpack-plugin');
module.exports = {
// ...
optimization: {
minimizer: [new TerserPlugin()],
},
plugins: [
new CompressionPlugin(),
],
};
2. Code Splitting
Split your code into smaller chunks to load only what’s necessary for each page, reducing initial load times.
// webpack.config.js
module.exports = {
// ...
optimization: {
splitChunks: {
chunks: 'all',
},
},
};
3. Tree Shaking
Utilize tree shaking to eliminate dead code and reduce bundle size.
// webpack.config.js
module.exports = {
// ...
optimization: {
usedExports: true,
},
};
4. Cache Busting
Implement cache busting techniques to ensure users always get the latest version of your assets.
// webpack.config.js
module.exports = {
// ...
output: {
filename: '[name].[contenthash].js',
},
};
Continuous Integration and Deployment
Automate your build and deployment process with continuous integration (CI) tools like GitHub Actions or Travis CI. Set up pipelines to run tests, build your application, and deploy it to production automatically whenever changes are pushed to your repository.
# .github/workflows/main.yml
name: CI/CD
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Install dependencies
run: npm install
- name: Run tests
run: npm test
- name: Build
run: npm run build
- name: Deploy
uses: jamesgeorge007/github-pages-deploy-action@releases/v3
with:
ACCESS_TOKEN: ${{ secrets.ACCESS_TOKEN }}
BRANCH: gh-pages
FOLDER: build
Conclusion
Optimizing your React build workflow is essential for delivering high-quality applications efficiently. By following the best practices outlined in this article, you can streamline your development process, improve your application’s performance, and automate deployment, ensuring a smooth transition from prototype to production. Keep experimenting with new tools and techniques to further enhance your workflow and stay ahead in the ever-evolving landscape of web development. Happy coding!