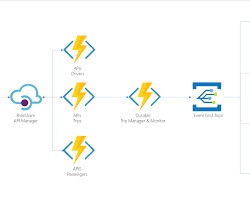
Serverless architecture has revolutionized the way we build and deploy applications. With its promise of reduced operational complexity and automatic scaling, serverless architecture has become a go-to for modern web applications. React.js, a popular JavaScript library for building user interfaces, pairs exceptionally well with serverless architecture, offering a powerful combination for developers.
In this guide, we’ll explore how to build a serverless application using React.js, covering the fundamentals, setting up the environment, coding examples, and best practices. Let’s dive in!
Table of Contents
1.Introduction to Serverless Architecture
2.Why Use Serverless with React.js?
3.Setting Up Your Development Environment
4.Building a Serverless React Application
a.Initializing a React App
b.Creating Serverless Functions
c.Integrating Serverless Functions with React
d.Deploying the Application
5. Best Practices for Serverless React Applications
6.Conclusion
1.Introduction to Serverless Architecture
Serverless architecture allows developers to build and run applications without managing the underlying infrastructure. In a serverless model, cloud providers dynamically manage the allocation of machine resources. Key components of serverless architecture include:
- Function as a Service (FaaS): Deploy individual functions that are executed in response to events.
- Backend as a Service (BaaS): Use third-party services to handle backend functionality like databases, authentication, and storage.
Benefits of Serverless Architecture
- Cost Efficiency: Pay only for the compute time you consume.
- Scalability: Automatic scaling based on the demand.
- Reduced Operational Complexity: No need to manage servers, which reduces operational overhead.
2.Why Use Serverless with React.js?
React.js is designed to build dynamic and interactive user interfaces. When combined with serverless architecture, React.js can offer several advantages:
- Simplified Development: Focus on writing code without worrying about server management.
- Improved Scalability: Serverless functions can scale independently based on demand.
- Faster Time to Market: Quickly deploy and iterate on applications.
3. Setting Up Your Development Environment
Before we start building our serverless React application, let’s set up our development environment. You’ll need:
Node.js: Ensure you have Node.js installed.
AWS Account: We’ll use AWS Lambda and API Gateway for our serverless functions.
AWS CLI: Install and configure the AWS Command Line Interface.
Serverless Framework: A toolkit for deploying serverless applications.
Installing Node.js
Download and install Node.js from the Official Website.
Setting Up AWS CLI
Install the AWS CLI by following the instructions here. Configure it using:
aws configure
Installing Serverless Framework
Install the Serverless Frame globally:
npm install -g serverless
4. Building a Serverless React Application
Let’s break down the process of building a serverless React application into manageable steps.
Initializing a React App
First, create a new React application using Create React App:
npx create-react-app serverless-react-app
cd serverless-react-app
Creating Serverless Functions
We’ll use the Serverless Framework to create and deploy our serverless functions. Initialize a new Serverless service:
serverless create --template aws-nodejs --path serverless-functions
cd serverless-functions
npm init -y
npm install
Update the ‘serverless.yml’ file to define a simple function:
service: serverless-react-app
provider:
name: aws
runtime: nodejs14.x
region: us-east-1
functions:
hello:
handler: handler.hello
events:
- http:
path: hello
method: get
Create a ‘handler.js’ file to define our function:
'use strict';
module.exports.hello = async (event) => {
return {
statusCode: 200,
body: JSON.stringify({
message: 'Hello from Serverless!',
}),
};
};
Deploy the function:
serverless deploy
After deployment, note the endpoint URL provided by the Serverless Framework.
Integrating Serveless Functions with React
In your React application, create a new service to interact with the serverless function. Create a ‘services’ directory and add an ‘api.js’ file:
// src/services/api.js
const API_ENDPOINT = 'YOUR_API_ENDPOINT_HERE';
export const fetchGreeting = async () => {
const response = await fetch(`${API_ENDPOINT}/hello`);
const data = await response.json();
return data;
};
Update the React Component to use this service:
// src/App.js
import React, { useEffect, useState } from 'react';
import { fetchGreeting } from './services/api';
function App() {
const [greeting, setGreeting] = useState('');
useEffect(() => {
const getGreeting = async () => {
const result = await fetchGreeting();
setGreeting(result.message);
};
getGreeting();
}, []);
return (
<div className="App">
<header className="App-header">
<h1>{greeting}</h1>
</header>
</div>
);
}
export default App;
Deploying the Application
To deploy the React application, we’ll use a service like Vercel, Netlify, or AWS Amplify. For this example, we’ll use Vercel.
Install the Vercel CLI:
npm install -g vercel
Deploy your React application:
vercel
Follow the prompts to complete the deployment. Your React application is now live and integrated with your serverless backend.
5.Best Practices forServerless React Applications
To ensure your serverless React application is robust, scalable, and maintainable, consider the following best practices:
Optimize Cold Start Performance
- Minimize Package Size: Reduce the size of your deployment package to improve cold start times.
- Provisioned Concurrency: For critical functions, use provisioned concurrency to keep instances warm.
Efficient API Design
- RESTful APIs: Design your APIs following REST principles for scalability and maintainability.
- GraphQL: Consider using GraphQL for flexible and efficient data fetching.
Security Best Practices
- Environment Variables: Store sensitive information in environment variables.
- IAM Roles: Use AWS IAM roles to grant least-privilege permissions to your functions.
Monitoring and Logging
- AWS CloudWatch: Use CloudWatch for logging and monitoring your serverless functions.
- Error Handling: Implement comprehensive error handling in your functions and client-side code.
Cost Management
- Resource Limits: Set resource limits on your functions to avoid unexpected costs.
- Analyze Usage: Regularly review your usage and adjust resources as necessary.
6 Conclusion
Building serverless applications with React.js offers a modern approach to web development, leveraging the scalability and cost-efficiency of serverless architecture with the power of React for building dynamic user interfaces. By following the steps outlined in this guide, you can create, deploy, and optimize a serverless React application effectively.
Serverless architecture simplifies the development process, allowing you to focus on writing code and delivering value to users without the burden of managing infrastructure. Embrace the serverless revolution and elevate your React.js applications to new heights. Happy coding!