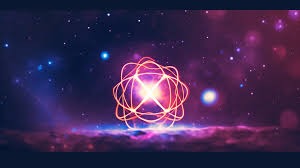
In the modern web development landscape, React and Astro have emerged as powerful tools for building efficient, scalable, and engaging websites. This article will explore how to use React with Astro, covering the basics, demonstrating with code, and providing insights to help you create stunning websites. Whether you’re a beginner or an experienced developer, this guide is for you!
What is React? π€
React is a popular JavaScript library for building user interfaces, particularly for single-page applications where you want a fast, interactive user experience. It allows developers to create reusable UI components.
Key features of React include:
- Component-Based Architecture: Build encapsulated components that manage their own state and compose them to make complex UIs.
- Virtual DOM: React updates the DOM efficiently by only changing what needs to be updated.
- Unidirectional Data Flow: Data flows down the component tree, making it easier to understand and debug.
What is Astro? π
Astro is a modern web framework for building fast, content-focused websites. It supports various front-end frameworks like React, Vue, and Svelte, allowing developers to choose the best tool for the job.
Key features of Astro include:
- Partial Hydration: Only the JavaScript needed for interactivity is loaded, leading to faster load times.
- Framework Agnostic: Use your preferred framework for components.
- Static Site Generation: Generates static HTML at build time, enhancing performance.
Why Combine React and Astro? π€
Combining React and Astro leverages the best of both worlds. React’s dynamic component-based architecture, paired with Astro’s performance optimizations, enables developers to create interactive and fast websites.
Getting Started π
Let’s dive into setting up a project with React and Astro. We’ll start by creating a new Astro project and then integrate React components into it.
Step 1: Create a New Astro Project
First, ensure you have Node.js installed. Then, create a new Astro project using the following commands:
npx create-astro@latest my-astro-react-project
cd my-astro-react-project
npm install
Step 2: Add React to Your Astro Project
Next, add React to your Astro project. Run the following command:
npm install @astrojs/react react react-dom
Now, configure Astro to use React by editing βastro.config.mjsβ:
import { defineConfig } from 'astro/config';
import react from '@astrojs/react';
export default defineConfig({
integrations: [react()],
});
Step 3: Create Your First React Component
Create a React component inside the βsrc/componentsβ directory. Let’s create a simple βHelloWorld.jsxβ component:
// src/components/HelloWorld.jsx
import React from 'react';
const HelloWorld = () => {
return (
<div>
<h1>Hello, World! π</h1>
<p>Welcome to your first React component in Astro!</p>
</div>
);
};
export default HelloWorld;
Step 4: Use Your React Component in an Astro Page
Now, you can use your React component in an Astro page. Create a new page at βsrc/pages/index.astroβ:
---
import HelloWorld from '../components/HelloWorld.jsx';
---
<html>
<head>
<title>My Astro React Project</title>
</head>
<body>
<HelloWorld />
</body>
</html>
Step 5: Run Your Project
Run your Astro project using the following command:
npm start
Visit βhttp://localhost:3000β to see your React component rendered in an Astro page. π
Advanced Example: Building a Blog π
Let’s take it a step further and build a simple blog with React components in an Astro project.
Step 1: Set Up Markdown Files for Blog Posts
Create a βpostsβ directory inside βsrcβ and add some Markdown files for blog posts:
// src/posts/first-post.md
---
title: 'First Post'
date: '2024-06-26'
---
This is the content of the first post.
// src/posts/second-post.md
---
title: 'Second Post'
date: '2024-06-27'
---
This is the content of the second post.
Step 2: Create a Blog Layout
Create a layout component for the blog
// src/layouts/BlogLayout.jsx
import React from 'react';
const BlogLayout = ({ children }) => {
return (
<div>
<header>
<h1>My Blog</h1>
</header>
<main>{children}</main>
<footer>
<p>Β© 2024 My Blog</p>
</footer>
</div>
);
};
export default BlogLayout;
Step 3: Create Blog Post Components
Create a component to display individual blog posts:
// src/components/BlogPost.jsx
import React from 'react';
const BlogPost = ({ post }) => {
return (
<article>
<h2>{post.title}</h2>
<p>{post.content}</p>
<p><em>{post.date}</em></p>
</article>
);
};
export default BlogPost;
Step 4: Fetch and Display Blog Posts in an Astro Page
Create a page to fetch and display the blog posts:
---
import { getCollection } from 'astro:content';
import BlogLayout from '../layouts/BlogLayout.jsx';
import BlogPost from '../components/BlogPost.jsx';
const posts = await getCollection('posts');
---
<BlogLayout>
{posts.map(post => (
<BlogPost key={post.slug} post={post} />
))}
</BlogLayout>
Step 5: Configure Astro to Use Content Collections
Add the following configuration to your βastro.config.mjsβ:
import { defineConfig } from 'astro/config';
import react from '@astrojs/react';
import mdx from '@astrojs/mdx';
export default defineConfig({
integrations: [react(), mdx()],
content: {
collections: {
posts: {
schema: {
title: 'string',
date: 'date',
},
},
},
},
});
Conclusion π
Congratulations! You have successfully created a website with React and Astro. You’ve learned how to set up a project, create and use React components, and build a simple blog.
React’s powerful UI capabilities combined with Astro’s performance optimizations make for an excellent developer experience. With this foundation, you can explore more advanced features and create even more dynamic and engaging websites.
Happy coding! π