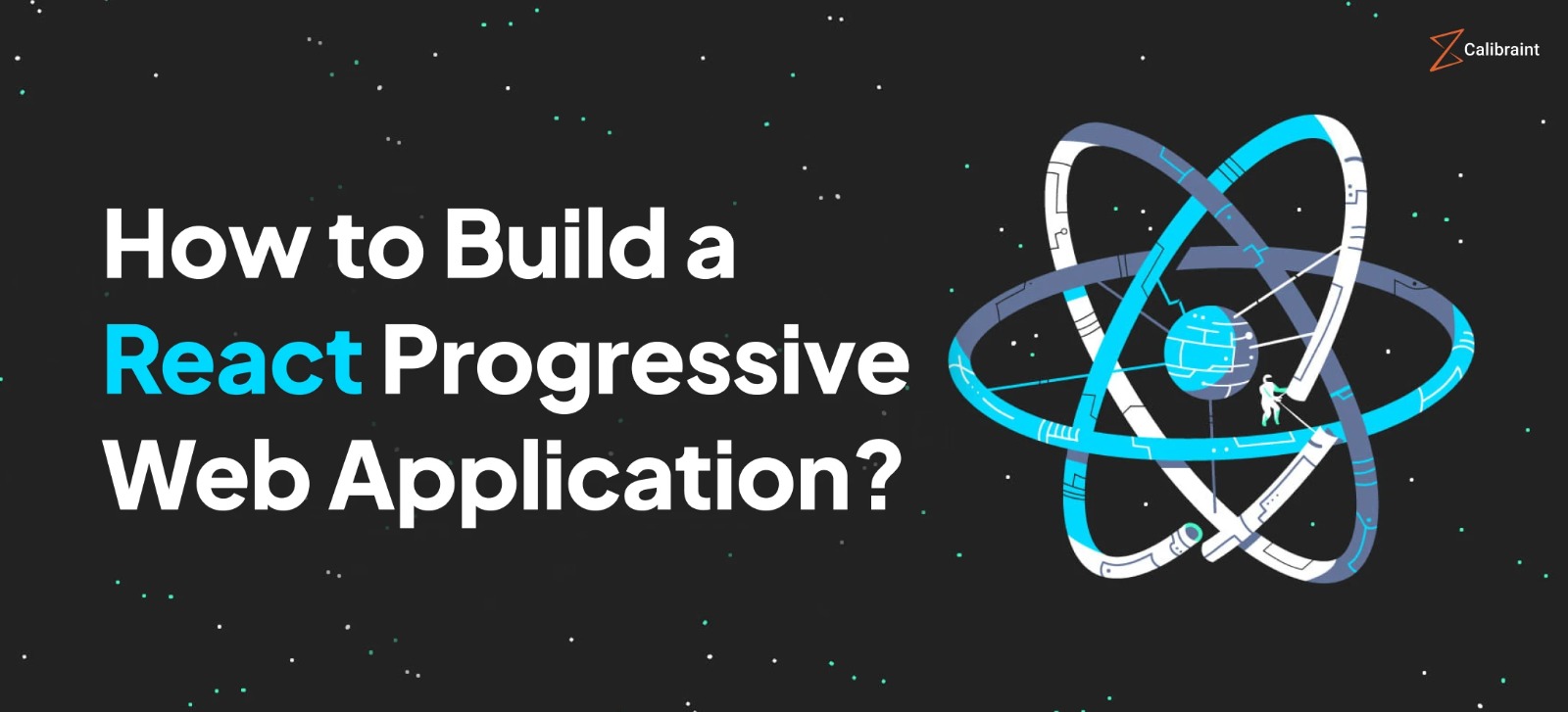
Progressive Web Apps (PWAs) are web applications that use modern web technologies to deliver app-like experiences to users. They combine the best of web and mobile apps, offering benefits such as offline capabilities, push notifications, and improved performance. In this article, we’ll explore how to build a PWA using React, a popular JavaScript library for building user interfaces.
Table of Contents
- Introduction to Progressive Web Apps
- Why Use React for PWAs?
- Setting Up Your React Project
- Converting a React App to a PWA
- Adding Service Workers
- Implementing Caching Strategies
- Enabling Push Notifications
- Testing and Deploying Your PWA
- Conclusion
1. Introduction to Progressive Web Apps
What is a Progressive Web App?
A Progressive Web App (PWA) is a type of application software delivered through the web, built using common web technologies including HTML, CSS, and JavaScript. They are intended to work on any platform that uses a standards-compliant browser, including both desktop and mobile devices. Key characteristics of PWAs include:
- Progressive: Works for every user, regardless of browser choice.
- Responsive: Fits any form factor, from desktop to mobile.
- Connectivity Independent: Enhanced with service workers to work offline or on low-quality networks.
- App-like: Feels like an app to the user with app-style interactions and navigation.
- Fresh: Always up-to-date thanks to the service worker update process.
- Safe: Served via HTTPS to prevent snooping and ensure content hasn’t been tampered with.
- Discoverable: Identifiable as an “application” thanks to W3C manifests and service worker registration, allowing search engines to find them.
- Re-engageable: Features like push notifications make it easy to re-engage with users.
- Installable: Users can keep apps they find most useful on their home screen without the hassle of an app store.
- Linkable: Easily share via URL and do not require complex installation.
Benefits of PWAs
- Improved Performance: Faster load times and smoother interactions.
- Increased Engagement: Features like push notifications and offline access increase user engagement.
- Cost-Effective: Easier and cheaper to develop and maintain compared to native apps.
- Broader Reach: Accessible on any device with a web browser, expanding your audience.
2. Why Use React for PWAs?
React is a JavaScript library developed by Facebook for building user interfaces. It is particularly well-suited for developing PWAs due to the following reasons:
- Component-Based Architecture: Makes it easy to manage and reuse code.
- Virtual DOM: Ensures efficient updates and rendering, improving performance.
- Large Ecosystem: A vast array of libraries and tools are available, making development faster and easier.
- Strong Community: A large and active community provides plenty of resources and support.
3. Setting Up Your React Project
To get started, you need to have Node.js and npm (Node Package Manager) installed on your machine. You can download them from Node.js official website.
Once you have Node.js and npm installed, you can create a new React project using Create React App, a comfortable environment for learning React and a great foundation for single-page applications.
npx create-react-app my-pwa
cd my-pwa
This command will set up a new React project in a directory named ‘my-pwa’.
4. Converting a React App to a PWA
Create React App provides a great starting point for creating a PWA out of the box. When you create a new project using Create React App, it already includes a service worker setup in the src directory. Let’s make sure our app is a PWA by following these steps:
Updating manifest.json
The manifest.json file provides metadata about your web application and is crucial for making your app installable. It’s located in the public directory. Here’s a sample configuration:
{
"short_name": "ReactApp",
"name": "Create React App Sample",
"icons": [
{
"src": "favicon.ico",
"sizes": "64x64 32x32 24x24 16x16",
"type": "image/x-icon"
},
{
"src": "logo192.png",
"type": "image/png",
"sizes": "192x192"
},
{
"src": "logo512.png",
"type": "image/png",
"sizes": "512x512"
}
],
"start_url": ".",
"display": "standalone",
"theme_color": "#000000",
"background_color": "#ffffff"
}
Registering the Service Worker
In the ‘src’ directory, locate the ‘index.js’ file. Ensure the service worker is registered by updating the ‘index.js’ file:
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import * as serviceWorkerRegistration from './serviceWorkerRegistration';
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById('root')
);
// Register the service worker for offline capabilities
serviceWorkerRegistration.register();
The ‘serviceWorkerRegistration.js’ file is included by default in the Create React App. It handles the registration of the service worker.
5. Adding Service Workers
Service workers are scripts that run in the background, separate from the web page, enabling features that don’t need a web page or user interaction. They are essential for PWAs because they provide offline capabilities, background syncs, and push notifications.
Here’s a basic service worker setup in the serviceWorker.js file:
const CACHE_NAME = 'my-pwa-cache-v1';
const urlsToCache = [
'/',
'/index.html',
'/static/js/bundle.js',
'/static/js/main.chunk.js',
'/static/js/vendors~main.chunk.js',
'/static/css/main.chunk.css',
'/static/media/logo.6ce24c58023cc2f8fd88fe9d219db6c6.svg',
// Add other assets you want to cache
];
self.addEventListener('install', event => {
event.waitUntil(
caches.open(CACHE_NAME).then(cache => {
return cache.addAll(urlsToCache);
})
);
});
self.addEventListener('fetch', event => {
event.respondWith(
caches.match(event.request).then(response => {
return response || fetch(event.request);
})
);
});
self.addEventListener('activate', event => {
const cacheWhitelist = [CACHE_NAME];
event.waitUntil(
caches.keys().then(cacheNames => {
return Promise.all(
cacheNames.map(cacheName => {
if (!cacheWhitelist.includes(cacheName)) {
return caches.delete(cacheName);
}
})
);
})
);
});
This script caches specified assets during the installation phase and serves them from the cache during fetch requests. It also handles cache updates during the activation phase.
6. Implementing Caching Strategies
Caching is a crucial part of making your PWA fast and reliable. There are different caching strategies you can implement depending on your use case.
Cache First Strategy
The cache first strategy tries to serve resources from the cache. If the resource is not available in the cache, it fetches it from the network.
self.addEventListener('fetch', event => {
event.respondWith(
caches.match(event.request).then(cachedResponse => {
return cachedResponse || fetch(event.request);
})
);
});
Network First Strategy
The network first strategy tries to fetch resources from the network. If the network is unavailable, it serves the resource from the cache.
self.addEventListener('fetch', event => {
event.respondWith(
fetch(event.request).catch(() => {
return caches.match(event.request);
})
);
});
Stale While Revalidate Strategy
The stale while revalidate strategy serves resources from the cache while fetching new resources from the network in the background.
self.addEventListener('fetch', event => {
event.respondWith(
caches.open(CACHE_NAME).then(cache => {
return cache.match(event.request).then(cachedResponse => {
const fetchPromise = fetch(event.request).then(networkResponse => {
cache.put(event.request, networkResponse.clone());
return networkResponse;
});
return cachedResponse || fetchPromise;
});
})
);
});
7. Enabling Push Notifications
Push notifications allow your PWA to send timely updates to users even when the app is not actively used. To implement push notifications, you’ll need a service like Firebase Cloud Messaging (FCM).
Setting Up Firebase
- Create a Firebase project at Firebase Console.
- Register your app with Firebase.
- Add the Firebase configuration to your project. Update your ‘public/index.html’ with the Firebase configuration:
<script src="https://www.gstatic.com/firebasejs/8.6.8/firebase-app.js"></script>
<script src="https://www.gstatic.com/firebasejs/8.6.8/firebase-messaging.js"></script>
<script>
// Your web app's Firebase configuration
var firebaseConfig = {
apiKey: "YOUR_API_KEY",
authDomain: "YOUR_AUTH_DOMAIN",
projectId
Conclusion
Building Progressive Web Apps (PWAs) with React is a powerful way to create fast, reliable, and engaging web applications. By leveraging React’s component-based architecture and the capabilities of service workers, you can enhance user experiences with offline functionality, push notifications, and improved performance. With tools like Create React App, setting up a PWA is straightforward, allowing developers to focus on delivering high-quality applications. By following the steps outlined in this article, you can start building your own PWA and take advantage of the benefits that PWAs offer to both developers and users.