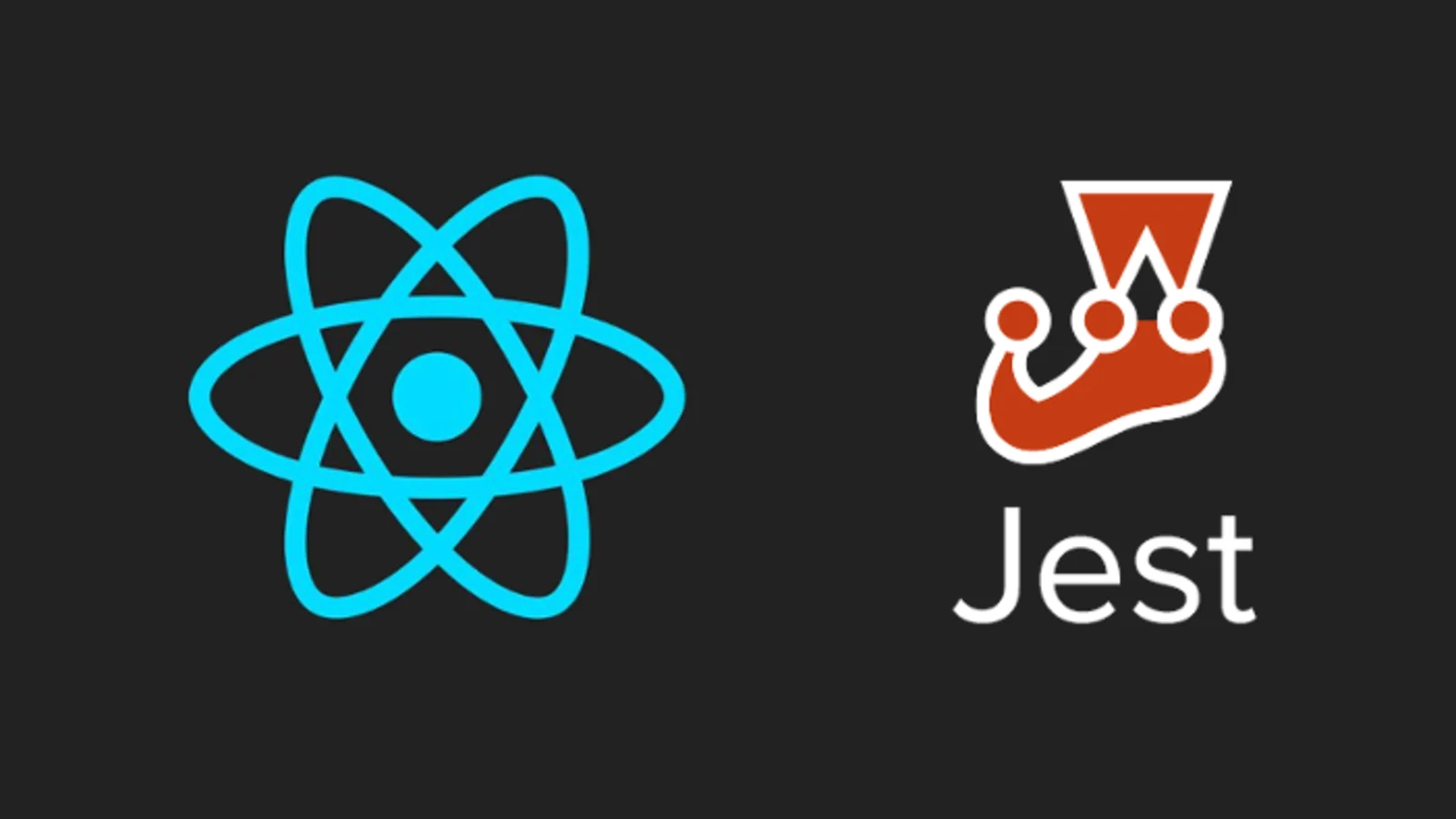
Introduction
Testing stands as a pivotal cornerstone in the development lifecycle, playing a decisive role in safeguarding the stability, dependability, and operational excellence of React components. This in-depth guide embarks on a journey through the utilization of Jest, a renowned testing framework for JavaScript, coupled with esteemed testing libraries like React Testing Library and Enzyme. Together, we will explore the intricate art of crafting diverse tests that meticulously validate the resilience and functionality of React components.
Why Testing is Essential
Before we dive into the technical details, let’s briefly discuss why testing is crucial. Testing helps catch bugs early in the development process, ensures that new features don’t break existing functionality, and provides a safety net for future code changes. By investing time in writing tests, developers can confidently make changes to the codebase, knowing that any regressions will be quickly identified.
Setting up jest and React Testing Library
To get started, let’s set up Jest and React Testing Library in a React project. Make sure you have Node.js and npm installed, and then run the following commands:
npm install --save-dev jest @testing-library/react @testing-library/jest-dom
Now,configure jest by adding a ‘jest.config.js’ file to the project root:
// jest.config.js
module.exports = {
testEnvironment: 'jsdom',
setupFilesAfterEnv: ['@testing-library/jest-dom/extend-expect'],
};
You’re now ready to write your first test.
Writing Unit Tests with jest
Unit tests focus on individual units of code, ensuring they work as expected in isolation. Let’s create a simple React component and write a unit test for it.
// MyComponent.jsx
import React from 'react';
const MyComponent = ({ value }) => {
return <div>{value}</div>;
};
export default MyComponent;
Now, let’s write a unit test for ‘ MyComponent ’ using jest:
// MyComponent.test.js
import React from 'react';
import { render } from '@testing-library/react';
import MyComponent from './MyComponent';
test('renders the component with the correct value', () => {
const { getByText } = render(<MyComponent value="Hello, Jest!" />);
expect(getByText('Hello, Jest!')).toBeInTheDocument();
});
This test ensures that the component renders correctly with the provided value.
Integration Testing with React Testing Library
Integration tests verify that different components work together as expected. Let’s create two components and write an integration test.
// ParentComponent.jsx
import React from 'react';
import ChildComponent from './ChildComponent';
const ParentComponent = ({ value }) => {
return (
<div>
<p>Parent Component</p>
<ChildComponent value={value} />
</div>
);
};
export default ParentComponent;
// ChildComponent.jsx
import React from 'react';
const ChildComponent = ({ value }) => {
return <p>{value}</p>;
};
export default ChildComponent;
Now ,let’s write an integration test for these components:
// ParentComponent.test.js
import React from 'react';
import { render } from '@testing-library/react';
import ParentComponent from './ParentComponent';
test('renders the parent and child components with the correct values', () => {
const { getByText } = render(<ParentComponent value="Integration Test" />);
expect(getByText('Parent Component')).toBeInTheDocument();
expect(getByText('Integration Test')).toBeInTheDocument();
});
This test ensures that the ‘ParentComponent’ and ‘ChildComponent’ render together correctly.
SnapShot Testing with jest
Snapshot testing captures the rendered output of a component and compares it to a stored snapshot. This is useful for detecting unintended changes in the UI.
Let’s modify our ‘ MyComponent ‘ test to include a snapshot test:
// MyComponent.test.js
import React from 'react';
import { render } from '@testing-library/react';
import MyComponent from './MyComponent';
test('renders the component with the correct value', () => {
const { getByText } = render(<MyComponent value="Hello, Jest!" />);
expect(getByText('Hello, Jest!')).toBeInTheDocument();
// Snapshot test
expect(getByText('Hello, Jest!')).toMatchSnapshot();
});
The first time you run this test, jest will generate a snapshot file.On subsequent runs,jest compares the current output with the stored snapshot,alerting you to any unexpected changes.
Testing with Enzyme
Enzyme is another popular testing utility for React. Let’s see how to use Enzyme for shallow rendering and component interaction testing.
First, install Enzyme:
npm install --save-dev enzyme enzyme-adapter-react-16 enzyme-to-json
Configure Enzyme in a setup file:
// setupTests.js
import { configure } from 'enzyme';
import Adapter from 'enzyme-adapter-react-16';
configure({ adapter: new Adapter() });
Now,let’s modify the ‘MyComponent.test.js’ file to use Enzyme:
// MyComponent.test.js
import React from 'react';
import { shallow } from 'enzyme';
import MyComponent from './MyComponent';
test('renders the component with the correct value', () => {
const wrapper = shallow(<MyComponent value="Hello, Enzyme!" />);
expect(wrapper.text()).toBe('Hello, Enzyme!');
});
Conclusion
In this comprehensive guide, we’ve covered the basics of testing React components using Jest and testing libraries. We explored unit testing, integration testing, snapshot testing, and briefly touched on testing with Enzyme. By incorporating these testing practices into your React development workflow, you can ensure the reliability and stability of your components, contributing to a more robust and maintainable codebase. Happy testing!