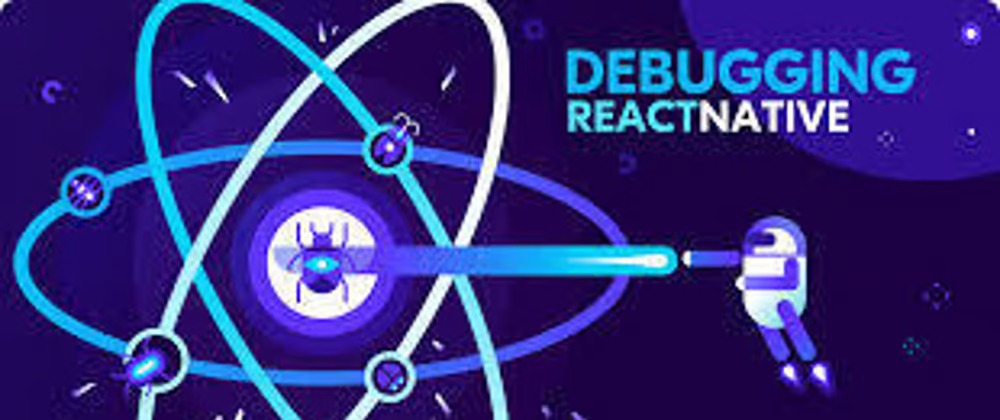
React Native is a popular framework for building mobile applications using JavaScript and React. However, debugging can often be challenging. This is where Reactotron comes in. Reactotron is a powerful tool that helps developers debug React Native applications effectively. In this article, we’ll dive deep into how to set up and use Reactotron for debugging your React Native applications. We’ll also explore some practical examples to illustrate its capabilities.
Table of Contents
- Introduction to Reactotron
- Setting Up Reactotron in Your React Native Project
- Configuring Reactotron
- Debugging with Reactotron
- Logging Messages
- Monitoring API Requests
- Tracking Redux Actions
- Handling Async Storage
- Custom Commands
- Best Practices for Debugging with Reactotron
- Conclusion
1. Introduction to Reactotron π
Reactotron is a desktop application for inspecting and debugging your React Native (and React JS) projects. It provides features like logging, state monitoring, API request tracking, and more. Reactotron makes it easier to visualize and interact with your app’s state and network requests, which can significantly speed up the debugging process.
2. Setting Up Reactotron in Your React Native Project π
To get started with Reactotron, you’ll need to install the Reactotron app on your desktop and the Reactotron library in your React Native project.
Step 1: Install Reactotron App
Download and install the Reactotron app from the official Reactotron website. It is available for macOS, Windows, and Linux.
Step 2: Install Reactotron Library
In your React Native project, install the Reactotron library and its dependencies:
npm install --save reactotron-react-native
npm install --save-dev reactotron-redux
npm install --save redux
3. Configuring Reactotron βοΈ
After installing the necessary packages, you need to configure Reactotron in your project. Create a new file named βReactotronConfig.jsβ in your project’s root directory and add the following code:
import Reactotron from 'reactotron-react-native';
import { reactotronRedux } from 'reactotron-redux';
import { AsyncStorage } from 'react-native';
// Initialize Reactotron
Reactotron
.setAsyncStorageHandler(AsyncStorage)
.configure() // controls connection & communication settings
.useReactNative() // add all built-in react native plugins
.use(reactotronRedux()) // add redux plugin
.connect(); // let's connect!
// Clear Reactotron on every time we load the app
if (__DEV__) {
Reactotron.clear();
}
// Making Reactotron available throughout the project
console.tron = Reactotron;
export default Reactotron;
Then, import and initialize this configuration in your βindex.jsβ or βApp.jsβ file:
import Reactotron from './ReactotronConfig';
if (__DEV__) {
import('./ReactotronConfig').then(() => console.log('Reactotron Configured'));
}
4. Debugging with Reactotron π
Now that Reactotron is set up, let’s explore its debugging features.
Logging Messages π
Reactotron can log messages, which can help you track the flow of your application:
console.tron.log('Hello, Reactotron!');
You can also log important variables:
const user = { name: 'John Doe', age: 30 };
console.tron.log(user);
Monitoring API Requests π
Reactotron can monitor network requests, which is essential for debugging API calls. To enable this, you need to use the βreactotron-apisauceβ plugin:
npm install --save reactotron-apisauce
npm install --save apisauce
Configure the plugin inβReactotronConfig.jsβ:
import apisauce from 'apisauce';
import Reactotron from 'reactotron-react-native';
import { reactotronRedux } from 'reactotron-redux';
import { reactotronApisauce } from 'reactotron-apisauce';
import { AsyncStorage } from 'react-native';
Reactotron
.setAsyncStorageHandler(AsyncStorage)
.configure()
.useReactNative()
.use(reactotronRedux())
.use(reactotronApisauce())
.connect();
if (__DEV__) {
Reactotron.clear();
}
console.tron = Reactotron;
export default Reactotron;
Then, create an API instance using βapisauceβ and log the requests:
import { create } from 'apisauce';
const api = create({
baseURL: 'https://jsonplaceholder.typicode.com',
});
api.addMonitor(response => {
console.tron.apisauce(response);
});
// Example API request
api.get('/users').then(response => {
console.tron.log(response.data);
});
Tracking Redux Actions π
Reactotron can track Redux actions and state changes, which is invaluable for debugging state management:
First, configure your Redux store to use Reactotron:
import { createStore, applyMiddleware, compose } from 'redux';
import rootReducer from './reducers';
import Reactotron from './ReactotronConfig';
const store = createStore(
rootReducer,
compose(applyMiddleware(...middleware), Reactotron.createEnhancer())
);
export default store;
Now, you can view Redux actions and state changes in the Reactotron app.
Handling Async Storage πΎ
Reactotron can inspect and modify Async Storage, making it easier to debug persistent storage:
import { AsyncStorage } from 'react-native';
// Set a value in Async Storage
AsyncStorage.setItem('user', JSON.stringify({ name: 'Jane Doe', age: 25 }));
// Get a value from Async Storage
AsyncStorage.getItem('user').then(user => {
console.tron.log(user);
});
Custom Commands β¨
Reactotron allows you to create custom commands for more specific debugging needs:
Reactotron.onCustomCommand({
title: 'Test Command',
description: 'A custom command to test something',
command: 'testCommand',
handler: () => {
console.tron.log('Test Command Executed');
}
});
5. Best Practices for Debugging with Reactotron π οΈ
To get the most out of Reactotron, consider the following best practices:
- Clear Logs Frequently: Use βReactotron.clear()β to keep your logs manageable.
- Leverage Custom Commands: Create custom commands for repetitive tasks.
- Monitor Performance: Use Reactotron to track performance issues in your app.
- Collaborate with Team Members: Share Reactotron logs and insights with your team to solve problems faster.
6. Conclusion π
Reactotron is a powerful tool that can greatly enhance your debugging experience in React Native. By leveraging its logging, network monitoring, state tracking, and custom command features, you can streamline your development workflow and build more robust applications. Start integrating Reactotron into your projects today and see the difference it makes!
Happy debugging! π