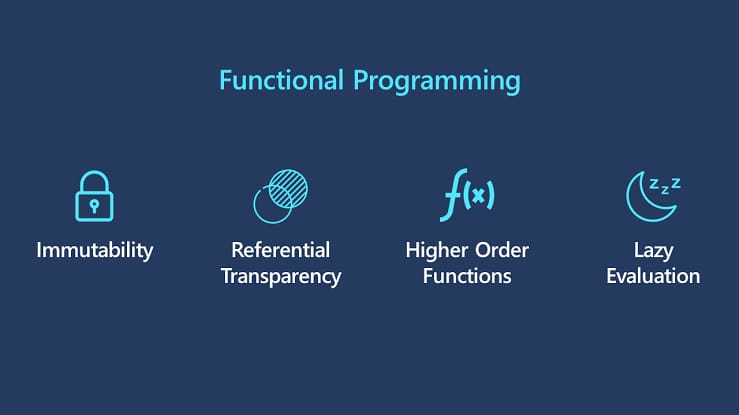
React has revolutionized the way web developers build user interfaces. With its component-based architecture and declarative syntax, React simplifies the process of creating dynamic and interactive web applications. However, as applications grow in complexity, managing state and side effects becomes increasingly challenging. This is where functional programming comes into play.
Functional programming (FP) is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data. Combining React with functional programming principles can lead to cleaner, more maintainable code. In this article, we’ll explore the fundamentals of functional programming and how they can be applied in React.
Understanding Functional Programming
Functional programming revolves around a few core concepts:
Immutability: Data is immutable, meaning once it’s created, it cannot be changed. Instead, new data is created from existing data.
Pure Functions: Functions that always return the same output for the same input and have no side effects.
Higher-Order Functions: Functions that take other functions as arguments or return functions as results.
Avoidance of Mutable State: Instead of mutating data, FP focuses on creating new data.
Now let’s see how these principles can be applied in React.
Immutability in React
Immutability plays a crucial role in React’s performance optimizations, particularly when it comes to efficiently updating the UI. By ensuring that data remains immutable, React can easily determine when to re-render components.
import React, { useState } from 'react';
const MyComponent = () => {
const [count, setCount] = useState(0);
const handleClick = () => {
// Avoid mutating state directly
setCount(count + 1); // This is a no-no!
// Instead, create a new state
setCount(prevCount => prevCount + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={handleClick}>Increment</button>
</div>
);
};
export default MyComponent;
In the above example, we’re using the ‘useState’ hook to manage state. Instead of mutating the ‘count’ directly, we’re using the ‘setCount’ function with the previous state to update it.
Pure Functions in React
Pure functions are essential in React because they ensure predictability and enable easier testing. In React, components should ideally be pure functions, meaning they only rely on their props and state.
import React from 'react';
const Greeting = ({ name }) => {
const greet = name => `Hello, ${name}!`;
return <div>{greet(name)}</div>;
};
export default Greeting;
In the above example, the ‘Greeting’ component is a pure function because it always returns the same output for the same input (‘name’).
Higher-Order Functions in React
Higher-order functions (HOFs) are functions that take other functions as arguments or return functions as results. In React, HOFs are often used to encapsulate common logic.
import React from 'react';
const withLogger = WrappedComponent => {
return class extends React.Component {
componentDidMount() {
console.log(`Component ${WrappedComponent.name} mounted.`);
}
render() {
return <WrappedComponent {...this.props} />;
}
};
};
const Greeting = ({ name }) => <div>Hello, {name}!</div>;
const GreetingWithLogger = withLogger(Greeting);
export default GreetingWithLogger;
In this example, ’ withLogger’ is a higher-order function that takes a component as an argument and returns a new component with logging functionality.
Avoiding Mutable State
Mutable state can lead to hard-to-debug issues in React applications, especially when dealing with asynchronous operations or complex state management. By embracing immutability, we can avoid many of these problems.
import React, { useState } from 'react';
const TodoList = () => {
const [todos, setTodos] = useState([]);
const addTodo = todo => {
setTodos(prevTodos => [...prevTodos, todo]);
};
return (
<div>
<ul>
{todos.map((todo, index) => (
<li key={index}>{todo}</li>
))}
</ul>
<button onClick={() => addTodo('New Todo')}>Add Todo</button>
</div>
);
};
export default TodoList;
In this example, we’re adding a new todo to the list without directly modifying the ‘todos’ array.
Conclusion
Incorporating functional programming principles into React can lead to cleaner, more maintainable code. By embracing immutability, pure functions, higher-order functions, and avoiding mutable state, developers can write more predictable and scalable React applications. As you continue to explore React and functional programming, remember to keep these principles in mind to build robust and efficient applications. Happy coding!