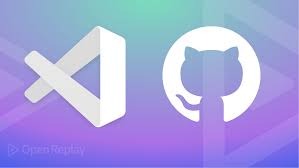
In this tutorial, you’ll learn how to develop a Visual Studio Code (VS Code) extension that can manage to-do lists across different programming languages. This extension will allow users to add, view, and navigate through to-do comments in their code, making task management easier for developers working with multiple languages. By the end of this guide, you’ll have a working extension that you can use and share with others.
Prerequisites
Before you start, ensure you have the following:
- Node.js and npm installed on your machine.
- Visual Studio Code installed.
- Basic knowledge of JavaScript and VS Code extensions.
Step 1: Set Up Your Extension Environment
1.Install VS Code Extension Generator:
Open your terminal and install the Yeoman generator for VS Code extensions:
npm install -g yo generator-code
2.Generate Your Extension:
Run the following command to start creating your extension:
yo code
Follow the prompts to configure your extension:
- Select “New Extension (TypeScript)” when asked for the type of extension.
- Provide a name for your extension, e.g., ‘multi-language-todo-manager’.
- Fill in other details like description, and confirm.
This command creates a new directory with all the necessary files to start developing your extension.
Step 2: Understanding the Generated Files
After generating the extension, you’ll find several files and folders in your project:
- ‘src/extension.ts’: This is the main file where you’ll write the logic for your extension.
- ‘package.json’: The configuration file for your extension, including commands, activation events, and dependencies.
- ‘src/test’: Contains test files for your extension.
Step 3: Define To-Do Commands in package.json
In ‘package.json’, you’ll define commands that will allow users to interact with the to-do manager. Add the following commands under the ‘contributes’ section:
"contributes": {
"commands": [
{
"command": "extension.addTodo",
"title": "Add To-Do"
},
{
"command": "extension.showTodos",
"title": "Show To-Dos"
}
]
},
"activationEvents": [
"onCommand:extension.addTodo",
"onCommand:extension.showTodos"
]
These commands will activate your extension when a user invokes them.
Step 4: Implement Command Logic in ‘extension.ts’
Open ‘src/extension.ts’ and implement the logic for adding and showing to-dos. Start by importing necessary modules:
import * as vscode from 'vscode';
Add To-Do Command
The ‘addTodo’ command will insert a to-do comment at the current cursor position:
function addTodo() {
const editor = vscode.window.activeTextEditor;
if (editor) {
const document = editor.document;
const position = editor.selection.active;
vscode.window.showInputBox({ prompt: 'Enter your to-do' }).then(todo => {
if (todo) {
const todoComment = `// TODO: ${todo}`;
editor.edit(editBuilder => {
editBuilder.insert(position, todoComment + '\n');
});
}
});
}
}
Show To-Do Command
The ‘showTodos’ command will search the document for to-do comments and display them in a list:
function showTodos() {
const editor = vscode.window.activeTextEditor;
if (editor) {
const document = editor.document;
const todoPattern = /(\/\/|#)\s*TODO: (.*)/g;
let todos: vscode.QuickPickItem[] = [];
for (let line = 0; line < document.lineCount; line++) {
const textLine = document.lineAt(line);
const matches = todoPattern.exec(textLine.text);
if (matches) {
todos.push({
label: matches[2],
detail: `Line ${line + 1}`,
description: matches[0]
});
}
}
vscode.window.showQuickPick(todos, { placeHolder: 'Your To-Dos' }).then(selected => {
if (selected) {
const line = parseInt(selected.detail!.replace('Line ', '')) - 1;
const position = new vscode.Position(line, 0);
editor.selection = new vscode.Selection(position, position);
editor.revealRange(new vscode.Range(position, position));
}
});
}
}
Step 5: Register Commands
Register these commands by modifying the ‘activate’ function in ‘extension.ts’:
export function activate(context: vscode.ExtensionContext) {
let addTodoCommand = vscode.commands.registerCommand('extension.addTodo', addTodo);
let showTodosCommand = vscode.commands.registerCommand('extension.showTodos', showTodos);
context.subscriptions.push(addTodoCommand);
context.subscriptions.push(showTodosCommand);
}
Step 6: Customizing the To-Do Pattern Matching
The regular expression in the ‘showTodos’ command is designed to match to-do comments in various languages. Here’s how it works:
const todoPattern = /(\/\/|#)\s*TODO: (.*)/g;
- ‘(\/\/|#)’: This part matches either ‘// ‘(used in languages like JavaScript, C++) or ‘#’ (used in languages like Python) as the comment symbol.
- ‘\s*TODO:’: This matches any amount of whitespace followed by “TODO:”.
- ‘(.*)’: This captures the actual to-do text following the “TODO:” marker.
If you want to support other comment styles (e.g., ‘<!– TODO:’ for HTML), you can modify the regular expression accordingly. Here’s an example that includes HTML-style comments:
const todoPattern = /(\/\/|#|<!--)\s*TODO: (.*)/g;
Step 7: Adding Configuration Options
To make your extension more customizable, you can add configuration options in ‘package.json.’ For example, let’s allow users to define their own to-do marker:
"contributes": {
"configuration": {
"type": "object",
"title": "Multi-Language To-Do Manager",
"properties": {
"todoMarker": {
"type": "string",
"default": "TODO",
"description": "Marker used to identify to-do comments."
}
}
}
}
You can then access this configuration in ‘extension.ts’:
const config = vscode.workspace.getConfiguration('multi-language-todo-manager');
const todoMarker = config.get('todoMarker', 'TODO');
const todoPattern = new RegExp(`(\/\/|#)\\s*${todoMarker}: (.*)`, 'g');
Step 8: Error Handling and Edge Cases
Ensure your extension handles potential errors gracefully:
- No To-Dos Found: If there are no to-dos found, inform the user.
if (todos.length === 0) {
vscode.window.showInformationMessage('No to-dos found in this file.');
}
User Cancels Input Box: If the user cancels the input box, do nothing.User Cancels Input
vscode.window.showInputBox({ prompt: 'Enter your to-do' }).then(todo => {
if (todo) {
// Insert to-do
}
});
Step 9: Testing and Debugging Your Extension
To test your extension:
- Press ‘F5’ in VS Code to open a new window with your extension loaded.
- Open a file, use ‘Ctrl+Shift+P’ to open the command palette, and type ‘Add To-Do’ or ‘Show To-Dos’.
- Add some to-dos and use the ‘Show To-Dos’ command to see them listed.
If your extension doesn’t work as expected, use ‘console.log’ statements or the VS Code debugger to troubleshoot.
Step 10: Packaging and Publishing Your Extension
1.Package Your Extension:
Install ‘vsce’, the tool used to package and publish VS Code extensions:
npm install -g vsce
Then, package your extension:
vsce package
This will generate a ‘.vsix ‘file that you can install locally or distribute.
2.Publish Your Extension: Follow the steps in the Visual Studio Code documentation to publish your extension to the VS Code Marketplace.
Step 11: Expanding the Extension
Here are some ideas for expanding your extension:
- Support for Additional Languages: Add support for more comment styles, such as /* ‘TODO’: for C-style languages or ‘<!– TODO:’ for HTML.
- Sidebar To-Do View: Create a custom view in the VS Code sidebar to display all to-dos in a project.
- Task Integration: Integrate with project management tools like Trello or GitHub Issues to sync to-dos.
- Mark as Completed: Add functionality to mark to-dos as completed or delete them directly from the list.
Step 12: Performance Considerations
For very large files, searching for to-dos can be resource-intensive. Consider optimizing your code by:
- Limiting the number of lines scanned in very large files.
- Processing to-dos in the background if necessary.
Conclusion
Congratulations! You’ve just created a multi-language to-do manager for VS Code. This extension allows developers to seamlessly manage their tasks across different programming languages within their code. With added features like customization, error handling, and performance optimizations, your extension is robust and ready for real-world use. You can continue to expand its functionality, integrating it with other tools or adding more advanced features. Happy coding!