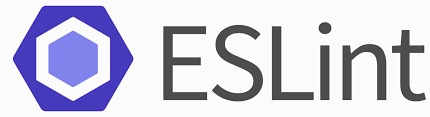
In the fast-paced world of web development, ensuring code quality, consistency, and maintainability is paramount. One indispensable tool in achieving these goals, particularly in React projects, is ESLint. ESLint is a powerful static code analysis tool that helps developers identify and fix problems in their JavaScript code. When coupled with React, ESLint becomes even more potent, offering a plethora of plugins and configurations tailored specifically for React development. In this comprehensive guide, we will delve deep into using ESLint with React, exploring its benefits, configuration options, and best practices.
Key Functionalities
Enforcing React-Specific Rules: The plugin enforces a comprehensive set of linting rules tailored for React development. These rules address common pitfalls and promote code quality by catching issues like:
- Incorrect JSX syntax
- Missing or misused props
- Unnecessary ReactDOM.render calls
- Deprecated React APIs
- Potential rendering performance bottlenecks
Preventing Errors: By identifying potential errors early in the development cycle, the plugin helps you avoid bugs that might otherwise surface during runtime, saving time and effort.
Maintaining Consistency: The plugin enforces coding conventions, ensuring a consistent style throughout your React project. This improves readability, maintainability, and collaboration among developers.
Catching Typos and Common Issues: The plugin goes beyond React-specific rules, catching common JavaScript errors and typos, further enhancing code quality.
Understanding Common Rules
The React ESLint plugin provides a rich set of rules, categorized into different severity levels:
Error: Rules that indicate potentially serious problems or syntax errors that prevent code from running correctly.
Warn: Rules that suggest potential issues or deviations from best practices but allow code to function.
Off: Rules that can be disabled if they conflict with your project’s style guide or preferences.
Here’s a breakdown of some essential rules:
- react/jsx-no-undef: Prevents using undefined React components.
- react/prop-types: Encourages the use of prop types for better type safety and documentation.
- react/jsx-no-duplicate-props: Catches duplicate props in JSX elements.
- react/jsx-uses-vars: Ensures that variables used in JSX are declared within the same scope.
- react/self-closing-comp: Promotes the use of self-closing components for readability.
- react/jsx-pascal-case: Enforces PascalCase for component names (configurable).
Tables of Contents:
- Introduction to ESLint
- Setting Up ESLint in a React Project
- Basic ESLint Rules for React
- Advanced ESLint Configurations for React
- Using ESLint Plugins for React
- Integrating ESLint with Code Editors
- Automating ESLint with Continuous Integration
- Conclusion
1. Introduction to ESLint:
ESLint is a JavaScript linting tool that helps developers detect and fix problems in their code. It enforces coding standards and best practices, ensuring consistency and readability across a codebase. ESLint works by parsing JavaScript code into an abstract syntax tree (AST) and then applying a set of rules to identify problematic patterns or code smells.
2.Setting Up ESLint in a React Project:
To begin using ESLint in a React project, you first need to install it along with any necessary plugins and configurations. You can do this using npm or yarn:
npm install eslint eslint-plugin-react --save-dev
yarn add eslint eslint-plugin-react --dev
Once installed, you can initialize ESLint configuration using the following command:
npx eslint --init
3.Basic ESLint Rules for React:
ESLint comes with a set of built-in rules that cover common JavaScript coding conventions. When working with React, it’s essential to enable rules specific to React development. Some basic ESLint rules for React include:
- ‘react/jsx-uses-react:’ Enforces that React is in scope.
- ‘react/jsx-uses-vars:’ Ensures that variables used in JSX are declared.
- ‘react/react-in-jsx-scope:’ Prevents common React errors.
4.Advanced ESLint Configurations for React:
In addition to basic rules, ESLint allows for advanced configurations tailored specifically for React projects. This includes rules for hooks, prop types, and JSX syntax. You can customize ESLint configurations by creating a ‘.eslintrc’ file in your project directory and specifying rules according to your preferences.
{
"extends": ["eslint:recommended", "plugin:react/recommended"],
"rules": {
"react/prop-types": "off",
"react-hooks/rules-of-hooks": "error",
"react-hooks/exhaustive-deps": "warn"
}
}
5.Using ESLint Plugins for React:
ESLint offers a wide range of plugins that extend its functionality for specific use cases. For React development, some popular ESLint plugins include:
- ‘eslint-plugin-react:’ Provides additional React-specific rules.
- ‘eslint-plugin-react-hooks:’ Offers rules for enforcing best practices with React hooks.
- ‘eslint-plugin-jsx-a11y:’ Ensures accessibility best practices are followed in JSX.
npm install eslint-plugin-react-hooks eslint-plugin-jsx-a11y --save-dev
6. Integrating ESLint with code Editors:
To streamline the development process, you can integrate ESLint with code editors such as Visual Studio Code, Sublime Text, or Atom. Most modern code editors offer extensions or plugins that automatically detect and highlight ESLint errors as you type.
7.Automating ESLint with Continuous Integration
In a collaborative development environment, it’s crucial to ensure that ESLint checks are performed consistently across all code changes. This can be achieved by integrating ESLint into your continuous integration (CI) pipeline. Tools like Travis CI, CircleCI, or GitHub Actions can be configured to run ESLint checks automatically whenever code is pushed to a repository.
8.Conclusion
In conclusion, ESLint is a valuable tool for maintaining code quality and consistency in React projects. By configuring ESLint with React-specific rules and plugins, developers can catch errors early, enforce best practices, and streamline the development process. With proper integration into code editors and CI pipelines, ESLint becomes an indispensable companion for React developers striving for excellence in their codebases.