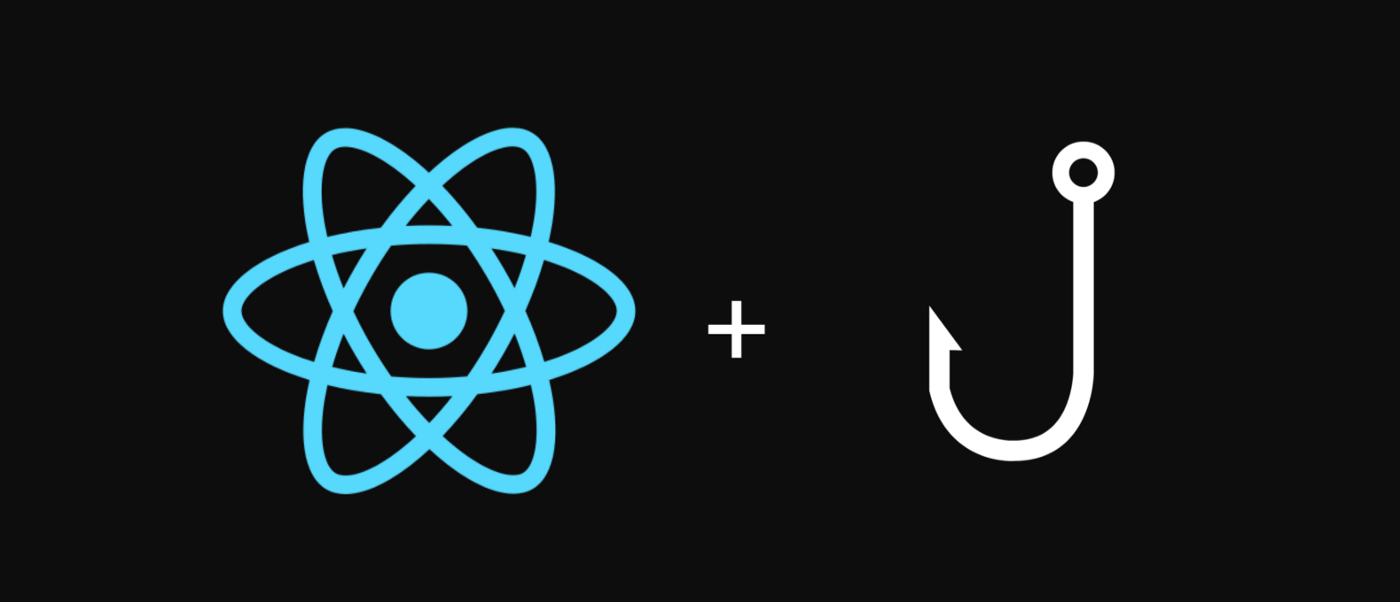
Introduction:
React Hooks has ushered in a new era of simplicity and efficiency in React application development. Among the multitude of features that Hooks offer, useState and useEffect stand out as crucial tools for managing state and handling side effects in functional components. In this comprehensive guide, we will explore the intricate relationship between React Hooks, components, and props. We will delve into best practices and advanced techniques to empower developers to create robust, modular, and maintainable React applications.
Understanding React Components:
React components are the cornerstone of user interfaces, serving as reusable building blocks that encapsulate both structure and behavior. Traditionally, components could be either functional or class-based. However, the advent of React Hooks elevated functional components by introducing state management capabilities.
React Props:
Props, short for properties, are a fundamental concept in React that facilitates the flow of data between components. They allow dynamic content rendering by passing data from a parent component to a child component. In class components, props are accessed via the this.props object, while in functional components, they are received as parameters.
// Functional Component
const Greeting = (props) => {
return <div>Hello, {props.name}!</div>;
};
// Usage
<Greeting name="John" />;
Command for Adding PropTypes:
Install prop-types:
npm install prop-types
This command installs the “prop-types” library for development dependency.
Import PropTypes in your component file:
import PropTypes from 'prop-types' ;
This line imports the”prop-types” library into your component file.
Define PropTypes for your component:
MyComponent.propTypes = {
name: PropTypes.string.isRequired,
age: PropTypes.number.isRequired,
};
Using this code you define PropTypes for your component,specifying the expected data types and whether they are required.
Using React Hooks with Functional Components:
React Hooks, specifically the useState hook, empowers functional components with the ability to manage state without the need for class components. Let’s explore a simple example:
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
Passing Props to Functional Components:
Functional components can easily receive and utilize props as parameters, enhancing modularity and reusability.
const UserProfile = (props) => {
return (
<div>
<p>Name: {props.name}</p>
<p>Age: {props.age}</p>
</div>
);
};
// Usage
<UserProfile name="Alice" age={25} />;
Combining Hooks and Props:
The real power of React emerges when Hooks and props are combined to create dynamic and interactive components. By using useState alongside props, developers can achieve a harmonious blend of local state management and external data integration.
const InteractiveCounter = ({ initialCount }) => {
const [count, setCount] = useState(initialCount);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
// Usage
<InteractiveCounter initialCount={5} />;;
Advanced Hook Patterns:
Explore advanced React Hooks patterns, including the use of custom hooks,useReducer,and useContext.These patterns provide sophisticated solutions for complex scenarios and contribute to cleaner,more maintainable code.
Custom Hooks
Custom hooks encapsulate logic for reuse across components,promoting modularity and readability.For example:
const useCustomHook = (initialState) => {
const [state, setState] = useState(initialState);
// Custom logic and side effects
return [state, customFunction];
};
// Usage
const [customState, customFunction] = useCustomHook(initialValue);
useReducer:
The useReducer hook is ideal for managing state logic in a more controlled and scalable manner:
const reducer = (state, action) => {
switch (action.type) {
case 'INCREMENT':
return { count: state.count + 1 };
// Handle other actions
default:
return state;
}
};
const CounterWithReducer = () => {
const [state, dispatch] = useReducer(reducer, { count: 0 });
return (
<div>
<p>Count: {state.count}</p>
<button onClick={() => dispatch({ type: 'INCREMENT' })}>Increment</button>
</div>
);
};
useContext:
The useContext hook simplifies the management of global state across components:
const UserContext = React.createContext();
const UserProvider = ({ children }) => {
const [user, setUser] = useState(null);
return (
<UserContext.Provider value={{ user, setUser }}>
{children}
</UserContext.Provider>
);
};
const UserProfileWithContext = () => {
const { user, setUser } = useContext(UserContext);
return (
<div>
<p>Name: {user.name}</p>
<button onClick={() => setUser({ name: 'New Name' })}>Change Name</button>
</div>
);
};
Error Handling with React Hooks:
Address strategies for error handling in functional components using error boundaries, the useEffect hook, and componentDidCatch in class components. Show how to gracefully manage errors to enhance user experience.
const ErrorBoundary = ({ children }) => {
const [error, setError] = useState(null);
useEffect(() => {
// Error handling logic
}, [error]);
if (error) {
return <p>Something went wrong!</p>;
}
return children;
};
// Usage
<ErrorBoundary>
<YourComponent />
</ErrorBoundary>
Testing React Components with Hooks:
Highlight the importance of testing and guide readers on testing components that use hooks. Introduce popular testing libraries like Jest and React Testing Library, demonstrating how to write effective unit tests.
import { render, fireEvent } from '@testing-library/react';
import YourComponent from './YourComponent';
test('increments count when button is clicked', () => {
const { getByText } = render(<YourComponent />);
const incrementButton = getByText('Increment');
fireEvent.click(incrementButton);
expect(getByText('Count: 1')).toBeInTheDocument();
});
Best Practices:
- Keep Components Small and Focused:
Break down complex components into smaller, more focused ones, each responsible for a specific part of the UI or functionality.
- Use Props for Data Flow:
Leverage props for passing data between components, promoting a unidirectional data flow and making components more predictable.
- Memoization for Performance:
Utilize the React.memo higher-order component to memoize functional components, preventing unnecessary renders when props remain unchanged.
- Custom Hooks for Reusability:
Extract common logic into custom hooks to enhance code reusability across multiple components.
Conclusion:
React Hooks, combined with effective prop usage, has revolutionized the development landscape, providing developers with powerful tools to create modular, efficient, and scalable React applications. By mastering the intricacies of React Hooks and adopting best practices, developers can navigate the ever-evolving world of web development with confidence, creating applications that are not only functional but also maintainable in the long run.