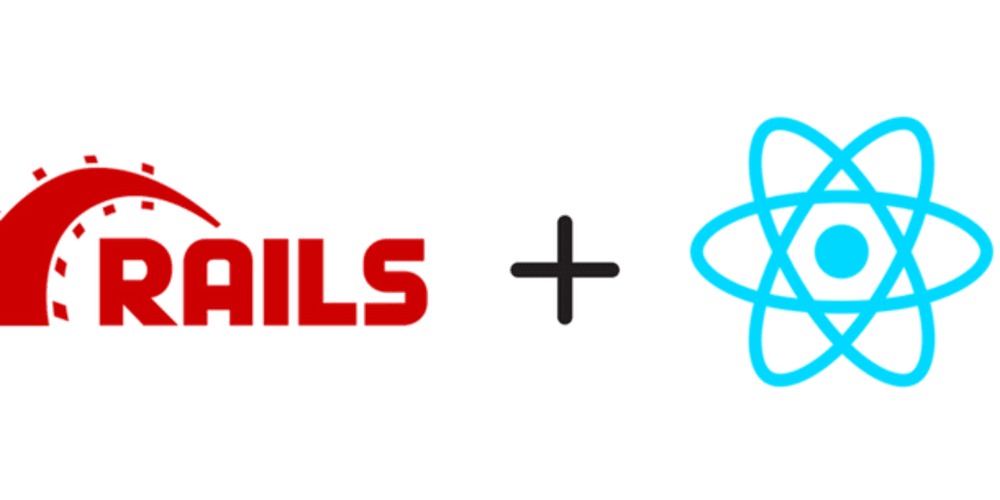
In the ever-evolving landscape of web development, the marriage of robust frontend frameworks with powerful backend technologies is crucial for creating modern, dynamic, and responsive applications. One such dynamic duo that has gained prominence is the combination of React and Ruby on Rails. This article takes a comprehensive look at the integration of React on Rails, exploring the strengths of each technology and showcasing how the amalgamation of these two powerhouses can significantly enhance the development process.
Understanding React: The Frontend Powerhouse
React, developed and maintained by Facebook, is a JavaScript library that has revolutionized the way developers build user interfaces. Its popularity stems from its declarative, component-based architecture, allowing for the creation of modular and reusable UI components.
The Core Features of React:
Component-Based Architecture: React breaks down the UI into reusable components, each encapsulating its logic and UI, promoting a modular and maintainable code structure.
Virtual DOM: React uses a virtual representation of the DOM, which is a lightweight copy of the actual DOM. This virtual DOM allows for efficient updates and minimizes unnecessary re-rendering, leading to enhanced performance.
JSX Syntax: JSX is a syntax extension for JavaScript that allows developers to write HTML within JavaScript code. This provides a more readable and concise way to describe the structure of UI components.
Unidirectional Data Flow: React follows a unidirectional data flow, ensuring that changes in the state of a component result in a predictable update of the user interface.
React has become a staple in frontend development due to its efficiency, flexibility, and vibrant community support.
The Rails Advantage: A Backend Powerhouse
On the server side, Ruby on Rails (Rails) has been a dominant force in web development since its introduction. Conceived with the principle of convention over configuration, Rails emphasizes developer happiness and productivity. Its opinionated structure, combined with a set of conventions, encourages best practices and accelerates development.
Key Features of Ruby on Rails:
Convention Over Configuration: Rails eliminates the need for developers to specify configurations by relying on conventions. This allows for a more streamlined development process and reduces the cognitive load on developers.
Active Record: Rails includes an Object-Relational Mapping (ORM) system called Active Record, simplifying database interactions and providing an intuitive way to work with databases.
RESTful Architecture: Rails promotes the use of RESTful principles, making it easy to build scalable and maintainable APIs. This architectural style enhances the predictability and scalability of web applications.
Scaffolding and Generators: Rails comes equipped with powerful scaffolding and generators, automating the creation of common components such as models, controllers, and views. This accelerates the development process and ensures consistency.
The combination of convention-driven development, the power of Active Record, and the focus on RESTful principles makes Ruby on Rails an ideal choice for building scalable and maintainable backend systems.
React on Rails
The integration of React within a Rails application, often referred to as React on Rails, capitalizes on the strengths of both technologies. React handles the frontend responsibilities, providing a rich and interactive user experience, while Rails manages the backend logic, routing, and database interactions. This synergy allows developers to harness the full potential of a comprehensive and efficient development stack.
Key Advantages of React on Rails:
Isomorphic Rendering:One of the standout features of React on Rails is isomorphic rendering, also known as server-side rendering (SSR). This approach allows the same codebase to be used for rendering components on both the server and the client. SSR enhances performance and search engine optimization (SEO) by delivering pre-rendered content to the client.
Hot Module Replacement (HMR): React on Rails supports Hot Module Replacement, a development feature that allows developers to see the changes they make in real-time without a full page reload. This accelerates the development cycle, making it faster and more efficient.
Scalability: The modular nature of React components aligns well with Rails’ emphasis on convention and modularity. This combination facilitates scalability, enabling development teams to manage and scale their applications more efficiently as the project grows.
Community Support: Both React and Rails boast vibrant communities with a wealth of resources. The integration of the two technologies benefits from the collective expertise of developers worldwide. A plethora of libraries, gems, and components are readily available, streamlining the development process.
Setting Up React on Rails
Setting up React on Rails is a straightforward process, thanks to the dedicated gem called react_on_rails. This gem provides generators and conventions that simplify the integration of React components into a Rails application.
Step 1: Install the react_on_rails gem
Add the gem to your Rails application’s Gemfile:
ruby
gem 'react_on_rails'
Install it using Bundler:
bundle install
Step 2: Run the Generator
Run the generator to set up the React infrastructure:
rails generate react_on_rails:install
This generator creates the necessary files and configurations to seamlessly integrate React into your Rails application.
Step 3: Create React Components
Create React components within the app/assets/javascripts/components directory. For example:
import React from 'react';
const HelloWorld = () => {
return (
<div>
<h1>Hello, World!</h1>
</div>
);
};
export default HelloWorld;
Step 4: Render React Components
Render React components within your Rails views using the react_component helper:
<!-- app/views/pages/home.html.erb -->
<%= react_component('HelloWorld') %>
By following these steps, you’ve successfully integrated React components into your Rails application.
Leveraging the Synergy: Practical Example
Let’s dive into a practical example to showcase the synergy between React and Rails. Imagine you’re building a task management application where users can create, update, and delete tasks. The React components handle the frontend interaction, while Rails manages the backend logic and database operations.
Rails Backend
#Ruby
class TasksController < ApplicationController
before_action :set_task, only: [:show, :update, :destroy]
def index
@tasks = Task.all
render json: @tasks
end
def show
render json: @task
end
def create
@task = Task.new(task_params)
if @task.save
render json: @task, status: :created
else
render json: @task.errors, status: :unprocessable_entity
end
end
def update
if @task.update(task_params)
render json: @task
else
render json: @task.errors, status: :unprocessable_entity
end
end
def destroy
@task.destroy
head :no_content
end
private
def set_task
@task = Task.find(params[:id])
end
def task_params
params.require(:task).permit(:title, :description, :completed)
end
end
This Rails controller provides the CRUD operations for tasks,interacting with the Task model.
React Frontend
Let’s create a React component for displaying and managing tasks.
import React, { useState, useEffect } from 'react';
const TaskList = () => {
const [tasks, setTasks] = useState([]);
const [newTask, setNewTask] = useState('');
useEffect(() => {
fetch('/tasks')
.then(response => response.json())
.then(data => setTasks(data))
.catch(error => console.error('Error fetching tasks:', error));
}, []);
const addTask = () => {
fetch('/tasks', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ title: newTask, completed: false }),
})
.then(response => response.json())
.then(data => setTasks([...tasks, data]))
.catch(error => console.error('Error adding task:', error));
setNewTask('');
};
const deleteTask = (taskId) => {
fetch(`/tasks/${taskId}`, {
method: 'DELETE',
})
.then(() => setTasks(tasks.filter(task => task.id !== taskId)))
.catch(error => console.error('Error deleting task:', error));
};
return (
<div>
<h2>Task List</h2>
<ul>
{tasks.map(task => (
<li key={task.id}>
{task.title}
<button onClick={() => deleteTask(task.id)}>Delete</button>
</li>
))}
</ul>
<div>
<input
type="text"
value={newTask}
onChange={(e) => setNewTask(e.target.value)}
/>
<button onClick={addTask}>Add Task</button>
</div>
</div>
);
};
export default TaskList;
In this React component,we fetch the list of tasks from the Rails backend, display them,and provide functionality to add and delete tasks.
Integrating React Component in Rails View
Now, let’s render this React component within a Rails view.
<!-- app/views/pages/tasks.html.erb -->
<%= react_component('TaskList') %>
By rendering the ‘TaskList’ React component within a Rails view, you seamlessly integrate frontend functionality into your Rails application.
Advanced Concepts: Isomorphic Rendering and Hot Module Replacement
Isomorphic Rendering (Server-Side Rendering)
React on Rails provides the ability for isomorphic rendering, allowing components to be rendered on both the server and the client. This can significantly improve the initial load time and SEO of your application.
import React from 'react';
const HelloWorld = () => {
return (
<div>
<h1>Hello, World!</h1>
</div>
);
};
export default HelloWorld;
To enable server-side rendering for this components,you need to specify it in the ‘react_component’ helper:
<!-- app/views/pages/home.html.erb -->
<%= react_component('HelloWorld', {}, { prerender: true }) %>
This will render the component on the server and send the pre-rendered HTML to the client.
Hot Module Replacement (HMR)
Hot Module Replacement is a development feature that allows you to see the changes you make in real-time without a full page reload. This can significantly speed up the development cycle.
React on Rails supports HMR out of the box. Simply run your Rails server with the following command:
rails s
And start your webpack server with:
./bin/webpack-dev-server
Now, any changes you make to your React components will be reflected in the browser without requiring a manual refresh.
Conclusion
React on Rails presents a formidable combination for building modern, scalable, and maintainable web applications. The seamless integration of React’s frontend capabilities with Rails’ robust backend functionality unlocks a world of possibilities for developers. Whether you’re creating a single-page application or a complex web platform, React on Rails empowers you to deliver high-quality, performant, and scalable solutions.
As the landscape of web development continues to evolve, the synergy between React and Rails remains a compelling choice for development teams seeking a comprehensive and efficient stack. By understanding the strengths of each technology and how they complement each other, you can harness the full potential of React on Rails and elevate your web development endeavors to new heights.