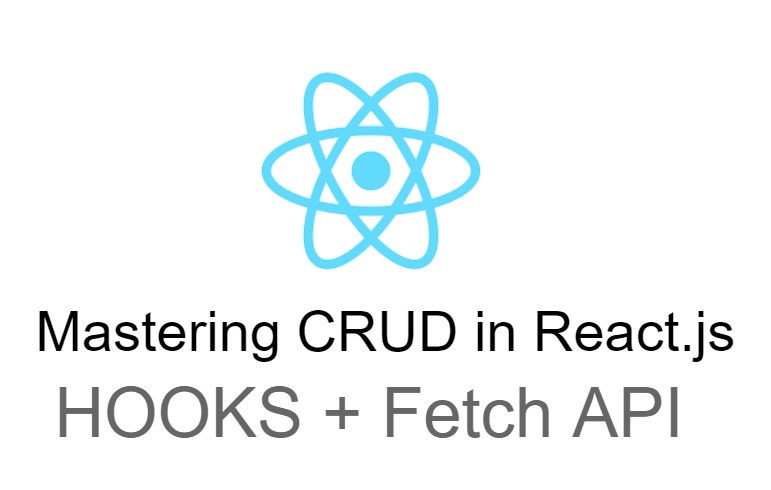
It’s no doubt, React Hooks have revolutionized the way developers manage state and lifecycle in React applications. Combined with the Fetch API, developers can implement powerful CRUD (Create, Read, Update, Delete) operations within their applications seamlessly.
In this comprehensive guide, we will dive deep into building a CRUD lab using React Hooks and Fetch API. By the end of this tutorial, you’ll have a solid understanding of how to implement CRUD operations in your React projects efficiently.
Prerequisites:
Before we dive into the implementation, ensure you have the following prerequisites installed:
- Node.js and npm
- Basic understanding of React.js
- Familiarity with JavaScript ES6 syntax
Setting Up the Project:
Let’s kick things off by setting up our React project. Open your terminal and follow these steps:
Create a new React project using Create React App:
npx create-react-app react-hooks-crud-lab
Navigate to the project directory
cd react-hooks-crud-lab
Install Axios for making HTTP requests:
npm install axios
Once installed,you’re ready to start implementing the CRUD operations in your React application.
Creating Components:
We’ll create four components for our CRUD operations: Create, Read, Update, and Delete. Let’s start with the Create component.
Create Component:
Inside the src folder, create a new file named Create.js. Add the following code:
import React, { useState } from 'react';
import axios from 'axios';
const Create = () => {
const [data, setData] = useState({
title: '',
description: ''
});
const handleChange = (e) => {
setData({ ...data, [e.target.name]: e.target.value });
};
const handleSubmit = async (e) => {
e.preventDefault();
try {
await axios.post('API_ENDPOINT', data);
alert('Data created successfully!');
setData({ title: '', description: '' });
} catch (error) {
console.error('Error creating data:', error);
}
};
return (
<div>
<h2>Create Data</h2>
<form onSubmit={handleSubmit}>
<input
type="text"
name="title"
placeholder="Title"
value={data.title}
onChange={handleChange}
/>
<textarea
name="description"
placeholder="Description"
value={data.description}
onChange={handleChange}
></textarea>
<button type="submit">Submit</button>
</form>
</div>
);
};
export default Create;
Replace ‘API_ENDPOINT’ with the actual endpoint where you’ll be sending your HTTP requests.
Read Component:
Create a new file named Read.js and add the following code:
import React, { useState, useEffect } from 'react';
import axios from 'axios';
const Read = () => {
const [data, setData] = useState([]);
useEffect(() => {
fetchData();
}, []);
const fetchData = async () => {
try {
const response = await axios.get('API_ENDPOINT');
setData(response.data);
} catch (error) {
console.error('Error fetching data:', error);
}
};
return (
<div>
<h2>Read Data</h2>
<ul>
{data.map((item) => (
<li key={item.id}>
<strong>{item.title}</strong>: {item.description}
</li>
))}
</ul>
</div>
);
};
export default Read;
Update Component:
Create a new file named Update.js and add the following code:
import React, { useState } from 'react';
import axios from 'axios';
const Update = () => {
const [data, setData] = useState({
id: '',
title: '',
description: ''
});
const handleChange = (e) => {
setData({ ...data, [e.target.name]: e.target.value });
};
const handleSubmit = async (e) => {
e.preventDefault();
try {
await axios.put(`API_ENDPOINT/${data.id}`, data);
alert('Data updated successfully!');
setData({ id: '', title: '', description: '' });
} catch (error) {
console.error('Error updating data:', error);
}
};
return (
<div>
<h2>Update Data</h2>
<form onSubmit={handleSubmit}>
<input
type="text"
name="id"
placeholder="ID"
value={data.id}
onChange={handleChange}
/>
<input
type="text"
name="title"
placeholder="Title"
value={data.title}
onChange={handleChange}
/>
<textarea
name="description"
placeholder="Description"
value={data.description}
onChange={handleChange}
></textarea>
<button type="submit">Submit</button>
</form>
</div>
);
};
export default Update;
Delete Component:
Create a new file named Delete.js and add the following code:
import React, { useState } from 'react';
import axios from 'axios';
const Delete = () => {
const [id, setId] = useState('');
const handleChange = (e) => {
setId(e.target.value);
};
const handleSubmit = async (e) => {
e.preventDefault();
try {
await axios.delete(`API_ENDPOINT/${id}`);
alert('Data deleted successfully!');
setId('');
} catch (error) {
console.error('Error deleting data:', error);
}
};
return (
<div>
<h2>Delete Data</h2>
<form onSubmit={handleSubmit}>
<input
type="text"
name="id"
placeholder="ID"
value={id}
onChange={handleChange}
/>
<button type="submit">Delete</button>
</form>
</div>
);
};
export default Delete;
Replace ‘API_ENDPOINT’ with the actual endpoint where you’ll be sending your HTTP requests.
Implementing CRUD Operations:
Now that we have our components set up, let’s integrate them into our main App component.
Open the App.js file and replace its content with the following code:
import React from 'react';
import Create from './Create';
import Read from './Read';
import Update from './Update';
import Delete from './Delete';
const App = () => {
return (
<div>
<Create />
<hr />
<Read />
<hr />
<Update />
<hr />
<Delete />
</div>
);
};
export default App;
This will render all our CRUD components in the main App component.
Congratulations! You’ve successfully built a CRUD lab using React Hooks and the Fetch API. You’ve learned how to create, read, update, and delete data in your React applications efficiently. Feel free to extend this project by adding validations, error handling, or integrating it with a backend server.
Happy coding!