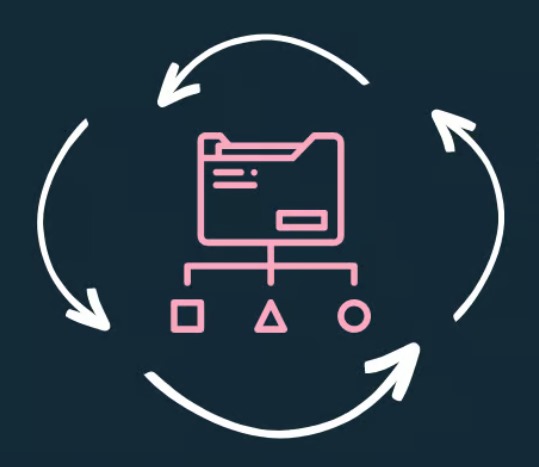
In the ever-evolving landscape of front-end development, React has emerged as a dominant force, offering developers a powerful and efficient way to build dynamic user interfaces. One of the most significant advancements in React’s evolution is the introduction of Hooks, a feature that allows developers to use state and other React features without writing a class. In this comprehensive guide, we’ll delve deep into React Hooks, focusing particularly on state management and event handling, empowering you to leverage these tools effectively in your projects.
Understanding React Hooks
Before we dive into state management and event handling with React Hooks, let’s briefly understand what Hooks are and why they are so significant.
What are React Hooks?
React Hooks are functions that let you use state and other React features in functional components. They were introduced in React 16.8 to solve various problems associated with class components, such as complex lifecycle methods, code reuse, and the difficulty of understanding how ‘this’ works in JavaScript. Hooks provide a more straightforward and more intuitive way to manage state and side effects in React components.
State Management with useState()
State management is a crucial aspect of building interactive user interfaces. React Hooks provide the ‘useState()’ hook, which allows functional components to manage state effortlessly. Let’s explore how to use ‘useState()’ effectively.
const [state, setState] = useState(initialState);
- ‘state’: The current state value.
- ‘setState’: Function to update the state.
- ‘initialState’: Initial value of the state.
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
Event Handling with useEffect()
Event handling is fundamental for building interactive user interfaces. React Hooks offer the ‘useEffect()’ hook, which enables you to perform side effects in functional components. Let’s see how we can handle events using ‘useEffect()’.
useEffect(() => {
// Effect code
return () => {
// Cleanup code (optional)
};
}, [dependencies]);
- ‘effect’:Function containing the side effect.
- ‘Dependencies’: Optional array of values that the effect depends on.
import React, { useState, useEffect } from 'react';
function MouseTracker() {
const [position, setPosition] = useState({ x: 0, y: 0 });
useEffect(() => {
const updateMousePosition = (e) => {
setPosition({ x: e.clientX, y: e.clientY });
};
window.addEventListener('mousemove', updateMousePosition);
return () => {
window.removeEventListener('mousemove', updateMousePosition);
};
}, []);
return (
<div>
<p>Mouse Position: {position.x}, {position.y}</p>
</div>
);
}
Combining State and Event Handling
Now that we understand how to manage state and handle events independently using React Hooks, let’s combine the two to create more complex and interactive components.
Example: Toggling a Component
import React, { useState } from 'react';
function Toggle() {
const [isOn, setIsOn] = useState(false);
return (
<div>
<button onClick={() => setIsOn(!isOn)}>
{isOn ? 'Turn Off' : 'Turn On'}
</button>
{isOn && <p>The component is on!</p>}
</div>
);
}
Conclusion
React Hooks have revolutionized the way developers approach state management and event handling in React applications. By providing a more concise and intuitive syntax, Hooks empower developers to write cleaner and more maintainable code. In this article, we explored how to use ‘useState()’ for state management and ‘useEffect()’ for event handling, along with practical examples to illustrate their usage. Armed with this knowledge, you’re now equipped to leverage the full power of React Hooks in your projects, unlocking new possibilities for building dynamic and interactive user interfaces. Happy coding!