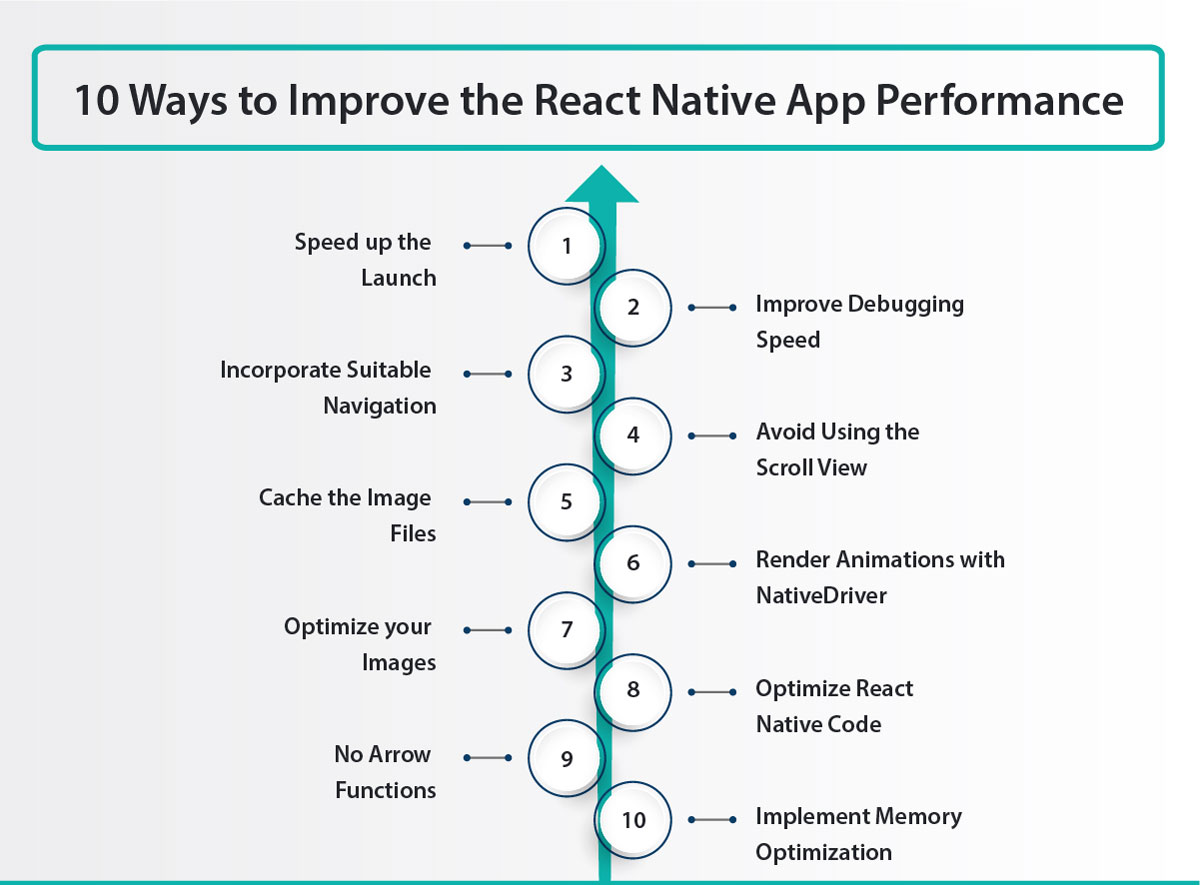
In today’s fast-paced digital world, mobile applications play a crucial role in our daily lives. Whether it’s for communication, productivity, entertainment, or business, mobile apps have become indispensable tools for billions of users worldwide. With the increasing complexity and demand for feature-rich applications, developers face the challenge of ensuring optimal performance while delivering a seamless user experience. This is where performance optimization becomes paramount, especially in frameworks like React Native.
Understanding React Native.
React Native, developed by Facebook, is a popular open-source framework for building cross-platform mobile applications. It allows developers to write mobile apps using JavaScript and React, sharing a single codebase across multiple platforms like iOS and Android. React Native’s component-based architecture and hot reloading feature enable rapid development and iteration cycles, making it a favorite among developers.
The Need for Performance Optimization.
While React Native offers numerous benefits for mobile app development, ensuring optimal performance remains a critical aspect of the development process. As applications grow in complexity and scale, performance bottlenecks can arise, leading to sluggish UI rendering, delayed interactions, and overall poor user experience. This is particularly evident on lower-end devices or in scenarios with poor network connectivity.
Impact on User Experience
The performance of a mobile application directly impacts user satisfaction and retention. Studies have shown that users are more likely to abandon apps that exhibit slow response times or frequent crashes. A smooth and responsive user interface not only enhances the perceived quality of an app but also fosters user engagement and loyalty. Therefore, optimizing performance is essential for delivering a competitive edge in the crowded app market.
Techniques for Performance Optimization
React Native provides developers with a variety of tools and techniques to optimize the performance of their applications. By implementing these best practices, developers can mitigate performance issues and deliver a superior user experience. Let’s explore some key strategies for performance optimization in React Native:
1.Lazy Loading
Lazy loading, also known as on-demand loading, is a technique that defers the loading of non-critical resources until they are actually needed. In the context of React Native, lazy loading can be applied to components, images, and data fetching operations. By only loading essential components initially and loading additional content as required, developers can reduce the initial load time of the application and improve overall performance.
import React, { lazy, Suspense } from 'react';
const LazyComponent = lazy(() => import('./LazyComponent'));
const App = () => (
<Suspense fallback={<LoadingSpinner />}>
<LazyComponent />
</Suspense>
);
2.Code Splitting
Code splitting involves breaking down the application code into smaller, more manageable chunks and loading them dynamically as needed. This helps reduce the initial bundle size of the application, resulting in faster load times and improved performance. React Native supports code splitting out of the box, allowing developers to split their code based on routes, features, or user interactions.
import { lazy } from 'react';
const LazyComponent = lazy(() => import('./LazyComponent'));
const App = () => (
<div>
<Suspense fallback={<LoadingSpinner />}>
<LazyComponent />
</Suspense>
</div>
);
3.Profiler Tool
The Profiler tool, introduced in React 16, provides valuable insights into the performance of React components. By measuring component render times, reconciliation, and other metrics, developers can identify performance bottlenecks and optimize their code accordingly. Integrating the Profiler tool into the development workflow enables real-time performance monitoring and helps ensure that the application meets performance goals.
import { Profiler } from 'react';
const onRender = (id, phase, actualDuration, baseDuration, startTime, commitTime, interactions) => {
console.log(`${id}'s ${phase} phase: ${actualDuration}ms`);
};
const App = () => (
<Profiler id="App" onRender={onRender}>
<MyApp />
</Profiler>
);
4.Image Optimization
Optimizing images is crucial for reducing the size of assets downloaded by the app. This includes using appropriate image formats (e.g., WebP for Android, JPEG or PNG for iOS), compressing images without sacrificing quality, and leveraging techniques like lazy loading and progressive image loading. By optimizing images, developers can significantly improve app performance, especially in scenarios where images play a prominent role, such as social media or e-commerce applications.
import { Image } from 'react-native';
const MyImage = () => (
<Image
source={{ uri: 'https://example.com/image.jpg' }}
resizeMode="cover"
style={{ width: 200, height: 200 }}
/>
);
5.Network Optimization
Efficient network usage is essential for ensuring fast data retrieval and minimizing latency in mobile applications. Developers can optimize network performance by reducing the number of HTTP requests, leveraging caching mechanisms (e.g., HTTP caching, AsyncStorage), and implementing strategies like data prefetching and batching. Additionally, choosing the appropriate networking libraries and protocols (e.g., HTTP/2, WebSocket) can further enhance network efficiency and responsiveness.
import axios from 'axios';
const fetchData = async () => {
try {
const response = await axios.get('https://api.example.com/data');
return response.data;
} catch (error) {
console.error('Error fetching data:', error);
return null;
}
};
Conclusion
In conclusion, performance optimization is a critical aspect of React Native development, essential for delivering fast, responsive, and engaging mobile applications. By employing techniques such as lazy loading, code splitting, and leveraging tools like the Profiler, developers can identify and address performance bottlenecks effectively. Moreover, optimizing images and network usage plays a vital role in ensuring efficient resource utilization and enhancing the overall user experience. As mobile apps continue to evolve and demand for high-performance experiences grows, prioritizing performance optimization will be key to success in the competitive app market.
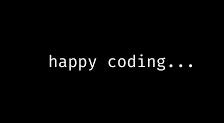